StreamReader and StreamWriter in C#
The System.IO namespace contains StreamReader and StreamWriter. Both classes come in handy when you need to read or write character-based data. These classes both focus on Unicode characters.
StreamReader and StreamWriter hail from the abstract class TextReader and textWriter.
StreamReader:
- A Stream Reader object represents a text reader that reads characters from a stream (much like a file).
- It facilitates character encoding and makes reading text from files easier.
StreamWriter:
- A text writer that writes characters to a stream, much like a file, is represented by a stream writer.
- It facilitates character encoding and makes writing text to files easier.
StreamReader
- You can read text from a stream and a file using the class StreamReader.
- You can read text from memory streams, network connections, and also file-based sources.
- Consider an example if it a gadget that allows us to open a book and read the pages one at a time.
Strings are read from streams using the StreamReader class in C#. It derives from the TextReader class. It offers the Read() and ReadLine() functions for reading data from the stream.
Example:
C# StreamReader that reads one line
using System;
using System.IO;
namespace MyNamespace
{
public class MyStreamReaderExample
{
public static void Main(string[] args)
{
FileStream fileStream = new FileStream("e:\\output.txt", FileMode.OpenOrCreate);
StreamReader reader = new StreamReader(fileStream);
string line = reader.ReadLine();
Console.WriteLine(line);
reader.Close();
fileStream.Close();
}
}
}
Output:
It prints the output.txt file content of the first line.
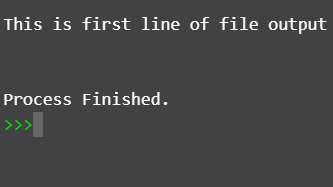
The "e:" drive's "output.txt" file is opened by the program using the FileStream class's FileMode.OpenOrCreate method. In other words, if the file is already exists, it can be opened for reading; otherwise, it will be created.
- The file's contents were read using the Stream Reader class. It also reads from the "output.txt" file because the FileStream object was uses when it was initialized.
- Using the ReadLine() method, the program reads the first line of the file and puts it in the line variable.
Note: The program will not print anything in to the console if the file is empty.
Example:
C# StreamReader that reads all lines
using System;
using System.IO;
namespace MyNamespace
{
public class MyStreamReaderExample
{
public static void Main(string[] args)
{
FileStream fileStream = new FileStream(filePath, FileMode.OpenOrCreate);
StreamReader reader = new StreamReader(fileStream);
string currentLine = "";
while ((currentLine = reader.ReadLine()) != null)
{
Console.WriteLine(currentLine);
}
reader.Close();
fileStream.Close();
}
}
}
Output:
It will print all the lines in the output.
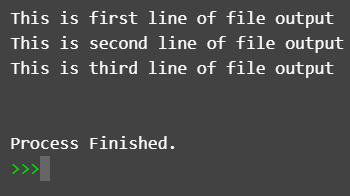
- File and sets its mode to FileMode using the FileStream class.OpenOrCreate. This mode guarantees that the file will be creates if it doesn't already exist and will be opened for reading.
- The FileStream object is then initialized using the StreamReader class so it may read the file's contents.
Stream Writer
- You can write data to a stream or a file using the C# class StreamWriter.
- It facilitates writing text to locations such as files or network connections.
- Think of it as a tool that lets your record ideas on paper and in a document.
- It can be use to write lines, characters, and content in to the new file.
Characters are written to a stream with a given encoding using the C# StreamWriter class. It comes from the class TextWriter. It has overloaded write() and write () functions for writing data into files.
Example of C# StreamWriter
StreamWriter class example that writes one line of data to a file.
using System;
using System.IO;
namespace MyNamespace
{
public class MyStreamWriterExample
{
public static void Main(string[] args)
{
string filePath = "D:\output.txt";
FileStream fileStream = new FileStream(filePath, FileMode.Create);
StreamWriter writer = new StreamWriter(fileStream);
writer.WriteLine("This is my modified StreamWriter example.");
writer.Close();
fileStream.Close();
Console.WriteLine("File created successfully...");
}
}
}
Output:
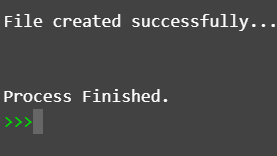
After rewriting the ”This is my modified StreamWriter example“ in a specific location, By setting its mode to FileMode, the FileStream class opens a file. Create. With this mode, the file will be creates if it doesn't already exist and truncated.
- The FileStream object is then uses to initialize the StreamWriter class, enabling it to write data to the file.
- The WriteLine() method of the StreamWriter is used to write this content StreamWriter sample into the file.
- The string is written using the WriteLine() method, followed by a newline character as the line terminator.
While StreamWriter is used to write data, writing down your thoughts into the paper StreamReader is used to read data, like reading a book. These classes are helpful for file input and output operations and reading and writing text data in C# applications.