Encapsulation in C#
Encapsulation is a concept in Object Oriented Programming Systems (OOPS). It depicts the combining of data and associated processes into one entity or unit.
Encapsulation helps to hide an object's internal state from the outside world and only reveals the necessary functions.
Declaring a class's fields (or data) private and making its manipulating methods (accessors and mutators) public are the ways by which encapsulation is achieved.
How is Encapsulation achieved in C#?
With the help of access modifiers and Properties, encapsulation is achieved.
Access Modifiers:
In c# 4, mail access modifiers are present. These are public, private, internal, and protected.
- Public:
Declaring methods, classes, or variables as public provides the accessibility of the code anywhere.
2. Private:
Declaring methods, classes, or variables as private ensures that the code is accessible only to the respected class members.
3. Protected:
Declaring methods, classes, or variables as protected ensures that the code is accessible only to the respected class members and derived class members.
4. Internal:
The code can only be accessed within the assembly. It cannot be accessed from another assembly.
Properties:
Properties include Getter and Setter methods, which allow encapsulation to be achieved. These techniques provide restricted access to a class's fields or underlying data.
Example to represent how Getter and Setter methods are used in encapsulation:
using System;
public class Example
{
private string name; // Private field
// Property with a getter and setter
public string Name
{
get
{
return name;
} // Getter method
set
{
// Ensure that the name is not null
if (!string.IsNullOrEmpty(value))
{
name = value;
}
else
{
Console.WriteLine("Name must not be empty");
}
} // Setter method
}
}
class Program
{
static void Main(string[] args)
{
// Create an instance of the Example class
Example example = new Example();
// Set the name using the setter method
example.Name = "Bebo";
// Get and print the name using the getter method
Console.WriteLine($"Name: {example.Name}");
// Try setting an empty name
example.Name = ""; // This will trigger the validation in the setter method
// Get and print the name again
Console.WriteLine($"Name: {example.Name}");
}
}
Output:
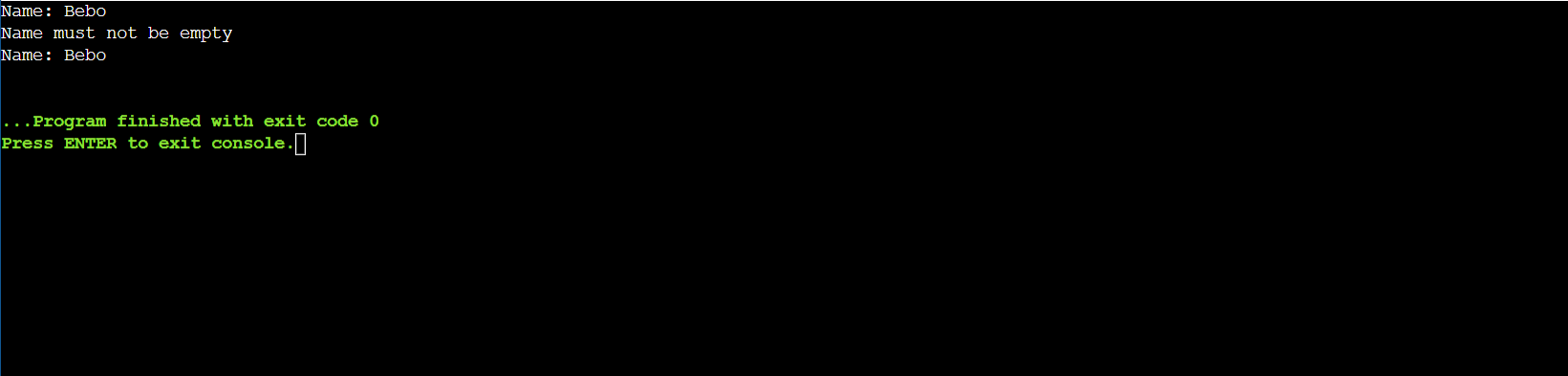
In the following C# code, encapsulation is accomplished in the "Example" class by declaring a private field called "name," which is accessible via a property called "Name." This property allows for controlled access to the class's internal state using getter and setter methods.
By retrieving the name field's value, the getter method makes sure that external code can access the data without having to change the underlying field directly. Concurrently, the setter method enforces restrictions like non-null and non-empty values and validates and assigns new values to the name.
The Main method demonstrates the usefulness of encapsulation in restricting access to class members and enforcing data restrictions by using the encapsulated property to set and retrieve the name value. Overall, the code demonstrates how encapsulation in C# programming promotes modularity, security, and maintainability.
Example Code in C# to show encapsulation:
using System;
public class Student
{
private string name;
private int student_id;
Private String gender;
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
public int Student_id
{
get
{
return student_id;
}
private set
{
student_id = value;
}
}
public String Gender;
{
get
{
return gender;
}
private set
{
gender = value;
}
}
public Student(string name, int student_id, String gender)
{
this.name = name;
This.student_id= student_id;
this.gender = gender;
}
public void DisplayStudentDetails()
{
Console.WriteLine($"Student Name: {Name}");
Console.WriteLine($"Student ID: {StudentId}");
Console.WriteLine($"Gender: {Gender}");
}
}
class Program
{
static void Main(string[] args)
{
// Creating a new student object
Student student1 = new Student("Alice", 1001, "Female");
// Displaying student details
Console.WriteLine("Student Details:");
student1.DisplayStudentDetails();
Student student2 = new Student("Bebo", 1002, "male");
student2.DisplayStudentDetails();
}
}
Output:
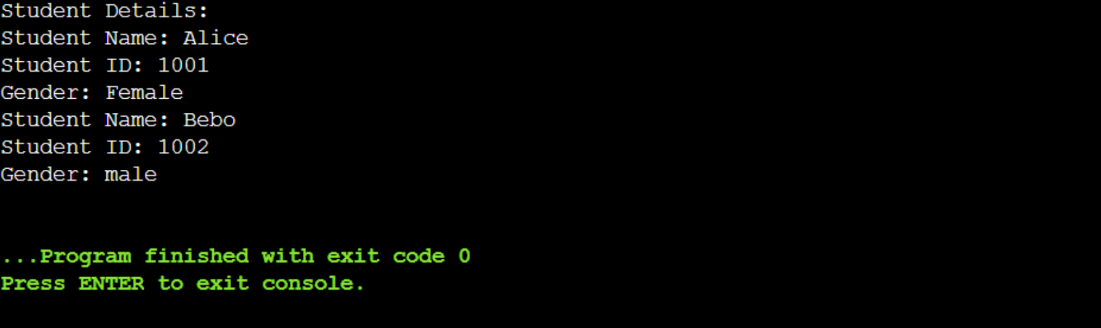
The provided code demonstrates the C # encapsulation. Using public properties, the "Student" class contains the student's name, ID, and gender in private fields. The constructor initializes these fields using values supplied as arguments. The student's data is printed out using the "DisplayStudentDetails()” function.
An instance of a "Student" class is created, and its details are shown in the Main method. Compilation errors occur when one tries to alter an encapsulated field outside of the class, demonstrating how encapsulation safeguards the internal state. By limiting access to class members and encouraging modularity, maintainability, and security in software development, encapsulation protects data integrity. It offers a tidy interface for external interaction while enabling the class to control its internal state.