REVERSE A STRING IN C#
INTRODUCTION
Programmers frequently need to reverse strings, which entails flipping the characters' orders. There are various ways to complete this work in C#, from simple and easy methods to more complex strategies. In this article, we'll look at a few alternative approaches to reversing strings in C# and offer clear, concise code samples to grasp the ideas at play better. There are two methods to reverse a String in C#.
Method 1: Using a character array
Converting a string of characters into a character array, working with the array, and then changing it back into a string are two frequent and effective ways to reverse a string in C#. With this method, we may directly access and change the order of specific characters within the string. The steps listed below can help us do this:
- Utilise the ToCharArray() function to transform the string into a character array.
- Set up two pointers, with the first pointing to the array's start and the second to its finish.
- Switch the characters at their appropriate pointers, inching up the start pointer and pushing down the end pointer as you go.
- Till the start pointer exceeds the end pointer, repeat step 3 as necessary.
- The ToString() function changes the updated character array into a string.
EXAMPLE
using System;
class Program
{
static void Main()
{
string input = "Javatpoint";
string reversed = ReverseString(input);
Console.WriteLine(reversed);
}
static string ReverseString(string input)
{
char[] charArray = input.ToCharArray();
int start = 0;
int end = charArray.Length - 1;
while (start < end)
{
char temp = charArray[start];
charArray[start] = charArray[end];
charArray[end] = temp;
start++;
end--;
}
return new string(charArray);
}
}
OUTPUT
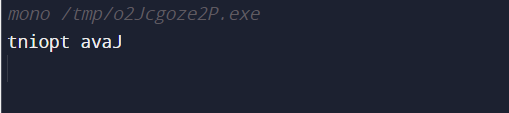
Method 2: Using StringBuilder
Utilizing the StringBuilder class, which has helpful string manipulation features, is another way to reverse a string in C#. In this method, the characters in the original string are iterated over, starting at the end, and are appended to a new StringBuilder object. Here's how to put it into practise:
- Construct a new instance of the StringBuilder class to store the inverted string.
- Repeat the original string's characters beginning at the end.
- Each character should be added to the StringBuilder object.
- Finally, the ToString() function changes the StringBuilder object into a string.
EXAMPLE
using System;
using System.Text;
class Program
{
static void Main()
{
string input = "Hello, World!";
string reversed = ReverseString(input);
Console.WriteLine(reversed);
}
static string ReverseString(string input)
{
StringBuilder reversedString = new StringBuilder();
for (int i = input.Length - 1; i >= 0; i--)
{
reversedString.Append(input[i]);
}
return reversedString.ToString();
}
}
OUTPUT
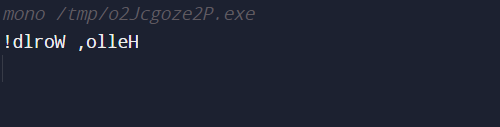
Method 3: Using LINQ
The LINQ (Language-Integrated Query) extension techniques are available in C# and enable quick and effective ways to manage data. By treating a string as a group of characters and using the Reverse() extension function, we can use LINQ to reverse a string.
EXAMPLE
using System;
using System.Linq;
class Program
{
static void Main()
{
string input = "Tutorial";
string reversed = ReverseString(input);
Console.WriteLine(reversed);
}
static string ReverseString(string input)
{
var reversedChars = input.Reverse();
string reversed = string.Concat(reversedChars);
return reversed;
}
}
OUTPUT

- The Reverse() extension function, which returns a string of characters in a different sequence, is the first thing we do in this method. The string is then used to merge these reversed elements into a new string, use the Concat() technique. We then give you the reversed string.
- We may reverse the strings in a clear and understandable way by using LINQ and its extension methods. The ReverseString method accepts a string as an input, uses LINQ to do the required transformations, and then returns the reversed text. By utilising the strength of LINQ and its creative syntax, this method offers a different way to reverse a string in C#.
CONCLUSION
In the first method, the string was transformed into a character array, the characters were switched about using pointers, and then the array was transformed back into a string. The second method built a reversed string by iterating through the characters in the initial string starting at the end using the StringBuilder class. Both strategies give sensible answers to the issue and several ways to get the desired outcome. The decision between the two strategies may be influenced by the problem's unique environment, personal preferences, and performance needs.