FileInfo Class in C#
A System.IO namespace in C# contains the FileInfo class, which provides information about and manipulates files. It represents a single file on the file system and gives you access to several properties and operations.
- FileInfo class in C# is used to manages and get data abouts files on the file system. It’s a member of the System.IO namespace.
- It represents a single file and offers several methods and properties that help to interact with it.
For example, you may read and write the file's content, verify its attributes, and carry out operations on the file, including copying, moving, and deleting.
Methods:
Methods | Description |
OpenRead() | You can read the file's contents using the method, which opens the file in read-only mode and returns a FileStream object. |
OpenWrite() | It can write data to the file using the FileStream object returned by the method, which opens the file in write mode. |
OpenText() | Opens the specified file for text reading and provides a StreamReader object that makes it easier to read text from the specified file. |
CopyTo(string destFileName) | The current file is copied to the destination path using the method. |
MoveTo(string destFileName) | The current file is transferred to the destination path using the method. |
Delete() | The method deletes the file that the FileInfo object is referring to. |
The FileInfo class can be used with these methods to interface with and manipulate files. These methods provide important functionality for managing files within your C# program, whether working with file contents, copying, moving, or deleting files.
- The FileStream class's Open function simplifies the process of starting a file opening by giving users the option to select a specific mode for the file action, decide on the access level (read, write, or both), and indicate the level of sharing that is authorized among other FileStream objects.
- A FileStream instance is created as a result of calling this function. The mode, access attributes, and sharing choices provided are reflected in this file instance.
- The operation's intended file mode (such as Open or Append) is specified by the mode argument.
- Whether the file should be accessible for reading, writing, or both depends on the access option.
- The share argument controls the type of access that other FileStream objects may have to the same file.
Properties:
- Name: Retrieves the file's name.
- FullName: Gets the files whole path using FullName.
- Directory: Retrieves a DirectoryInfo object that represents the file's parent directory.
- Exists: Gets a boolean value indicating whether the file exists or not.
- Length: Retrieves the file's length in bytes.
- CreationTime: Returns or sets the file's creation time.
- Last written: Gets or sets the most recent time the file was written.
- Attributes: Gets or modifies a file's attributes, such as read-only, hidden, etc.
Example:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\myfolder\newfile.txt"; // Specify the desired file path
// Create a FileInfo object
FileInfo fileInfo = new FileInfo(filePath);
try
{
// Check if the file already exists
if (!fileInfo.Exists)
{
// Create the file using the Create method
using (FileStream fs = file info.Create())
{
// You can perform any necessary operations using the FileStream fs
Console.WriteLine("File created successfully.");
}
}
else
{
Console.WriteLine("File already exists.");
}
}
Catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output:
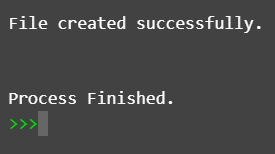
- The required file path is in a brand-new FileInfo object. The Exists property of the FileInfo object is then used to determine whether the file is present.
- We create the file using the Create() method if it doesn't already exist.
- When we finish the FileStream, the using statement ensures that it is appropriately disposed of.
Remember to substitute the desired location and filename where you wish to create the new file for "C:myfoldernewfile.txt."
Example:
In C#, you can copy an existing file to a different location and optionally give it a new name by using the CopyTo() function of the FileInfo class.
The CopyTo() method can be used as shown below:
using System;
using System.IO;
class Program2
{
static void Main()
{
string sourceFilePath = @"C:\myfolder\source.txt"; // Specify the path of the source file
string destinationFilePath = @"C:\myfolder\destination.txt"; // Specify the path of the destination file
// Create FileInfo objects for source and destination files
FileInfo sourceFileInfo = new FileInfo(sourceFilePath);
FileInfo destinationFileInfo = new FileInfo(destinationFilePath);
try
{
// Check if the source file exists
if (source file info. Exists)
{
// Copy the source file to the destination using the CopyTo method
FileInfo copiedFileInfo = sourceFileInfo.CopyTo(destination file path, true);
Console.WriteLine("File copied successfully to the destination.");
}
else
{
Console.WriteLine("Source file does not exist.");
}
}
Catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output:
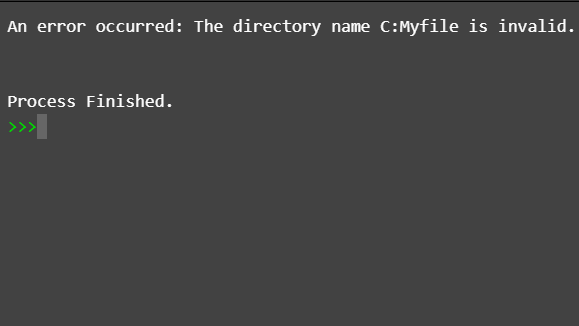
- Two FileInfo objects are being created in this example, one for the source and one for the destination files.
- We utilize the CopyTo() function on the source FileInfo object to copy the file to the designated destination.
- If the destination file already exists, the CopyTo() method's second argument truly permits overwriting.
- In "C:myfoldersource.txt" and "C:myfolderdestination.txt," replace "C:myfoldersource.txt" with the source file's path and the destination file's location and name, respectively.
Example:
A program to delete a file
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\myfolder\Some.txt"; // Specify the file path to delete
// Create a FileInfo object
FileInfo fileInfo = new FileInfo(filePath);
try
{
// Check if the file exists
if (file info. Exists)
{
// Delete the file using the Delete method
fileInfo.Delete();
Console.WriteLine("File deleted successfully.");
}
else
{
Console.WriteLine("File not found.");
}
}
Catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output:
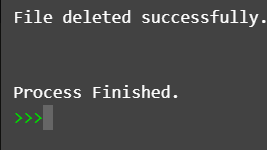
Using the given filePath, you build a FileInfo object with the name fileInfo.The Exists property of the fileInfo object determines whether the file is present within a try block.
- Change "C:myfolderfileToDelete.txt" in this program to the real location of the file you wish to remove.
- The Delete method of the FileInfo class will be used by the program when it is run to try and delete the specified file.
- The program shows the notification “File deleted successfully.” after removing the file from the particular folder. The program enters the else block and shows the message "File not found." if the file does not exist.
- Any exceptions that arise throughout the process such as file locks, permissions problems, etc.. are caught by the program in the catch block, and an error message that includes the exception's message to be displayed.
Example:
This program demonstrates how to deal with files using different FileInfo class methods and properties:
using System;
using System.IO;
using System.Security.AccessControl;
class Program
{
static void Main()
{
string filePath = @"C:\myfolder\sample.txt"; // Specify the file path
// Create a FileInfo object
FileInfo fileInfo = new FileInfo(filePath);
try
{
// Check if the file exists
if (!fileInfo.Exists)
{
// Create the file using the CreateText method and write some content
using (StreamWriter writer = fileInfo.CreateText())
{
writer.WriteLine("Hello, FileInfo!");
}
}
// Copy the file to a new location
string destination path = @"C:\myfolder\backup\sample_backup.txt";
fileInfo.CopyTo(destination path, true);
// Move the file to a different location
string newFilePath = @"C:\myfolder\newlocation\sample.txt";
fileInfo.MoveTo(newFilePath);
// Get file access control
FileSecurity fileSecurity = fileInfo.GetAccessControl();
// Display the file path
Console.WriteLine("File path: " + fileInfo.FullName);
// Display file size
Console.WriteLine("File size: " + fileInfo.Length + " bytes");
// Display file creation time
Console.WriteLine("Creation time: " + fileInfo.CreationTime);
// Display file last write time
Console.WriteLine("Last write time: " + fileInfo.LastWriteTime);
// Display file attributes
Console.WriteLine("Attributes: " + fileInfo.Attributes);
// Display file access control details
Console.WriteLine("Access control: " + file security);
Console.WriteLine("Program executed successfully.");
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output:
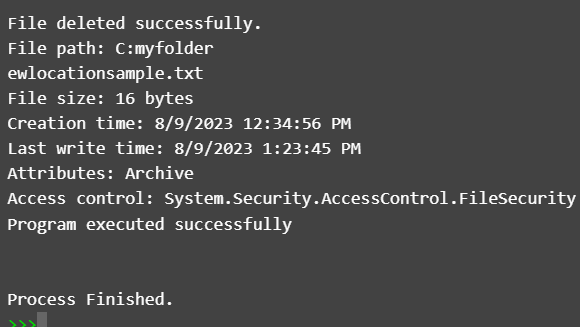
- For the given filePath, a FileInfo object is created. It utilizes the CreateText method for creating the file and write some content into it if it doesn't already exist it not creates.
- The CopyTo method is where used to show how to copy the file to a backup location.
- The MoveTo method uses the program to relocate the file for to a new location.
- Using the GetAccessControl method, it retrieves the access control settings.
- The program displays information about there file, including the file's path, size, creation and last write times, properties, and access control settings.
- These actions automatically catch and presents any exceptions as error messages.
Please be aware that depending on the actual execution environment and the paths you supply in the program, the values of file paths, timestamps, and characteristics will change. The output will show the data obtained through the program's use of FileInfo methods and properties.
s