BinaryReader and BinaryWriter in C#
In C#, the System.IO namespace provides classes like BinaryReader and BinaryWriter that let read and write binary data to and from files and streams. These classes come in handy when reading and writing non text files like photos, audio, and unique data formats and when you need to interact with raw binary data.
BinaryReader:
The System.IO namespace contains the BinaryReader class, which reads primitive data types from binary streams. With this tool, you can read binary data from a file and other stream and convert it back to a original data types.
- Integers, floating-point values, characters, byte arrays, and other formats of data can all be read using the multiple methods offered by the BinaryReader class.
- Binary (.bin) files were handled by the BinaryReader class.
- It reads binary values encoded in a certain way for primitive data types.
- Primitive data types can be read from streams more easily thanks to methods provided by the BinaryReader class.
Methods:
Method | Method signature | Description |
ReadBoolean: | Public virtual bool ReadBoolean() and ReadByte | Reads a single byte of Boolean data from the stream. |
ReadChar: | public virtual byte ReadByte(); | Reads one byte (one byte) from the stream. |
ReadInt16: | ReadChar(); | Reads a character from the stream (2 bytes long). |
ReadString: | public virtual string ReadString(); | Reads a string from the stream, as described. The length of the string is prefixed with a 7-bit integer encoded. |
ReadBytes | public virtual byte[] ReadBytes(int count). | Reads a certain number of bytes into a byte array from the stream. |
ReadSingle: | public virtual float ReadSingle(); | Reads a single-precision floating-point value of size 4 bytes from the stream. |
ReadDouble: | public virtual double ReadDouble(); | Reads a double-precision floating-point value in 8 bytes from the stream. |
ReadDecimal | public virtual ReadDecimal(); | Reads a 16-byte decimal value from the stream with the method signature |
These techniques let see read many kinds of data from a binary stream. When using BinaryReader, be careful to call the correct method based on the data type as our wish to read and confirm that the data format corresponds to the technique used to write the binary data.
Example:
using System;
using System.IO;
class Program1
{
static void Main()
{
string filePath = “data.bin”;
using (BinaryReader readers = new BinaryReader(File.Open(filePath, FileMode.Open)))
{
int intValue = reader.ReadInt32();
float floatValue = reader.ReadSingle();
byte[] byteArray = reader.ReadBytes(10); // Reads 10 bytes into a byte array.
// Process the read data here.
Console.WriteLine($"Read int value: {intValue}");
Console.WriteLine($"Read float value: {floatValue}");
Console.WriteLine($"Read byte array: {BitConverter.ToString(byteArray)}");
}
}
}
Output:
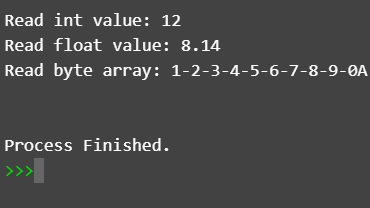
Using a BinaryReader, the program you gave reads binary data from a file called data.bin and interprets the data as particular data types.
Note: The contents of the “data.bin” file will determine the final product. The output will change appropriately if the file has different binary data or cannot be located.
The program reads binary data from a file called "data.bin" (you should change this to the path to your binary file) using the BinaryReader class from the System.IO namespace.
- The ReadBytes method is then used to read ten bytes into a byte array (byteArray). A Crucial NoteMake sure the "data.bin" file is present at the requested location or enter the correct file path.
- As the program reads particular data kinds (int, float, and bytes) from the file, ensure the binary file has data in the desired format. Incorrect data reading may produce unexpected results or problems.
BinaryWriter:
Primitive data types are written to a binary stream using the System.IO namespace BinaryWriter class. This class is quite helpful when dealing with non-text files and unique data formats and when creating or writing binary data to files or streams.
- The BinaryWriter class offers several ways to write various forms of data, including characters, integers, floating-point numbers, byte arrays, and many more.
- It stores the information in binary form, enabling you to store unprocessed, unencodes data.
Method | Description |
Write(String): | It uses the BinaryWriter's current encoding to write a length-prefixed string to the stream. Utilizes the encoded characters to advance the stream position. |
Write(float): | Stream is written with a four-byte floating-point value. The stream position is advanced by four bytes. |
Write(long): | Writes a signed integer of eight bytes to the stream. The stream position is advanced by eight bytes. |
Write(Boolean): | Writes a one-byte Boolean value to the stream (0 for false, 1 for actual). One byte is added to the stream location. |
Write(Byte): | An unsigned byte is written to the stream. One byte is added to the stream location. |
Write(Char): | Writes Unicode characters to the stream while taking the character encoding into account. Based on the characters written advances the stream position. |
Write(Decimal): | Creates a decimal value and writes it to the stream. The stream position is advanced by 16 bytes. |
Write(Double): | Writes a floating-point value of eight bytes to the stream. The stream position is advanced by eight bytes. |
Write(Int32): | Writes a signed integer of four bytes to the stream. The stream position is advanced by four bytes. |
The BinaryWriter class's write methods let you write various data types to a binary stream. Depending on the magnitude of the data being written, the methods advance the stream position. When working with binary data, consider the endianness and data size issues.
How Does the C# BinaryWriter Work?
The C# BinaryWriter class offers a simple method for writing binary data to a file or stream. It handles the binary representation of numerous data kinds and writes them consecutively to the output stream.
The BinaryWriter functions as follows:
Opening the Stream: The class opens the stream in write mode when you create an instance of BinaryWriter and give it a file path or stream. In other words, it prepares to write data to the designated file or stream.
Writing Data: Once the stream is open, you can write various sorts of data using one of the Write methods offered by the BinaryWriter class. For instance
writer.Write(07);
writer.Write(1.57f);
writer.Write(“T&E”);
writer.Write(true);
Position Advancement: The internal position of the stream is advanced by the specified number of bytes after each piece of data is written, depending on the type of data you wrote. For instance, writing an integer (Int32) moves the location forward by 4 bytes.
Closing and Flushing: It's crucial to close the BinaryWriter instance or use its Flush method after you've finished writing data. Doing this properly closes the stream, and any buffered data is written to the underlying stream.
Resource management: The BinaryWriter class implements IDisposable; therefore, to release resources and close the underlying stream, you should utilize it inside of a using statement or directly execute its Dispose method.
Endianness and Encoding: BinaryWriter writes binary data in the machine's native endianness, which on most Windows systems is little-endian. Additionally, the System's default encoding is utilized for string writing, which might not be appropriate for cross-platform compatibility. You must manually manage it if you require certain endianness or encoding.
- The BinaryWriter class handles stream management, write position advancement, and converting and writing various data types to their binary representations.
- It would help to be careful with endianness and encoding when working with data from various platforms or systems.
- It's a valuable tool when you need to work with binary data.
Example:
using System;
using System.IO;
class Program
{
static void Main()
{
String filePath = "D:\output.bin"; // Replace this with the path where you want to create the binary file.
using (BinaryWriter writer = new BinaryWriter(File.Open(filePath, FileMode.Create)))
{
int intValue = 42;
float floatValue = 3.14f;
byte[] byteArray = { 0x1, 0x2, 0x3, 0x4, 0x5 };
writer.Write(intValue);
writer.Write(floatValue);
writer.Write(byteArray);
}
Console.WriteLine("Binary data has been written to the file.");
}
}
Output:
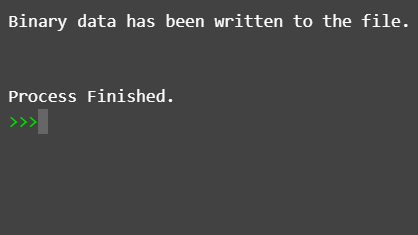
The integer, float, and byte array representations in binary form are all contained in the file.
Always supply a proper file path and exercise caution while handling binary data. When reading the data back with BinaryReader or another tool, ensure you understand it correctly.
Example:
Program to create a binary file
using System;
using System.IO;
namespace FileHandlingDemo
{
class Program3
{
static void Main(string[] args)
{
using (BinaryWriter writer = new BinaryWriter(File.Open("D:\\MyBinaryFile.bin", FileMode.Create)))
{
// Writing Error Log
writer.Write("0x80234400");
writer.Write("Windows Explorer Has Stopped Working");
writer.Write(true);
}
Console.WriteLine("Binary File Created");
Console.ReadKey();
}
}
}
Output:
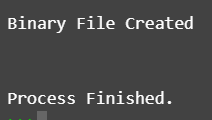
The "MyBinaryFile.bin" file on the D: drive is instantiated and connected to the BinaryWriter. Write mode (FileMode.Create) is used to open it. The string representing the hexadecimal error code "0x80234400"."Windows Explorer Has Stopped Working" is the error message in string form, confirmed as a Boolean value.
On the D: drive, the program creates a binary file called "MyBinaryFile.bin" and uses the BinaryWriter to write an error report, a message, and a boolean value to the file.