C# Sortedlist With Examples
A key or value pair collection sorted according to keys is called a SortedList in C#. It is part of a group that includes both generic and non-generic items .System.Collections.A generic namespace contains the definition of the generic Sorted List, while Sorted List that is not generic is specified under System. Here, we'll discuss the non-generic type Sorted List under the collection’s namespace.
Important Points:
- The IEnumerable, ICollection, IDictionary, and ICloneable interfaces are implemented by the SortedList class.
- A Sorted List entry can be retrieved using the key or the index.
- Duplicate keys are not permitted in SortedList.
- Due to the non-generic collection, SortedList allows you to store values of the same type and various types. It is required for the values to be the same type if you use a generic SortedList in your program.
- Since the compiler will raise an error, you cannot use the same SortedList to store keys for multiple data types. As a result, always include the key in the same kind of SortedList.
- You can also convert a SortedList's key/value pair into a DictionaryEntry.
How to create a Sorted List?
The SortedList class offers six distinct sorts of constructors; however, in this case, we only use the SortedList() constructor.
SortedList(): Each key added to the SortedList object and the IComparable interface has a starting capacity that is predetermined by default and is sorted.
Step 1: Use the keyword to include the System.Collections namespace in your program:
using System.Collections;
Step 2: Create a SortedList as shown below by utilizing the SortedList class:
SortedList list_name = new SortedList();
Step 3: There are three ways to get the SortedList's key/value pairs:
1. for loop
2. Using Index
3. foreach loop
1. for loop: Use a for loop to the SotedList’s value pairs.
Example:
for (int x = 0; x < my_slist1.Count; x++)
{
Console.WriteLine("{0} and {1}",
my_slist1.GetKey(x),
my_slist1.GetByIndex(x));
}
2. Using Index: The SortedList's value can be accessed using the index.
Example:
Console.WriteLine("Value is:{0}", my_slist1[1.04]);
string x = (string)my_slist[1.02];
Console.WriteLine(x);
3. foreach loop: To retrieve the key/value pairs in the SortedList, use a foreach loop.
Example:
foreach(DictionaryEntry pair in my_slist1)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
Example:
Program:
The CODE is as follows:
// C# program to illustrate how
// to create a sortedlist
using System;
using System.Collections;
class Preethi {
// Main Method
static public void Main()
{
// Creating a sortedlist
// Using SortedList class
SortedList my_slist1 = new SortedList();
// Adding key/value pairs in
// SortedList using Add() method
my_slist1.Add(1.02, "This");
my_slist1.Add(1.07, "Is");
my_slist1.Add(1.04, "SortedList");
my_slist1.Add(1.01, "Tutorial");
foreach(DictionaryEntry pair in my_slist1)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
Console.WriteLine();
// Creating another SortedList
// using Object Initializer Syntax
// to initialize sortedlist
SortedList my_slist2 = new SortedList() {
{ "b.09", 234 },
{ "b.11", 395 },
{ "b.01", 405 },
{ "b.67", 100 }};
foreach(DictionaryEntry pair in my_slist2)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
}
}
OUTPUT:
The Output for the following program will be:
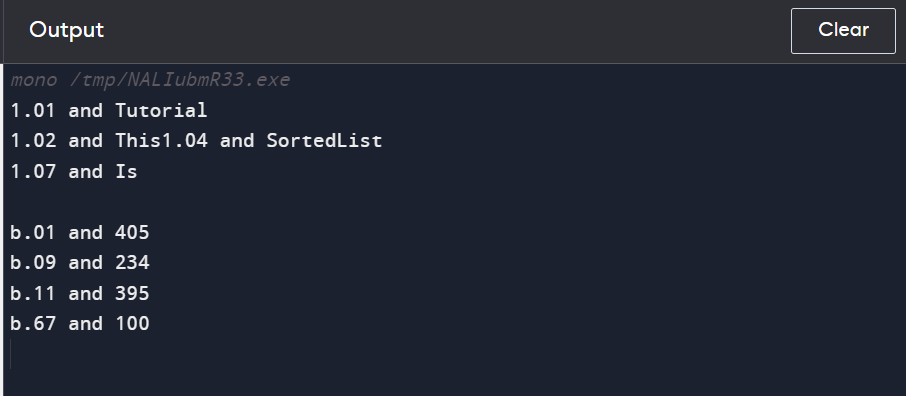
How to remove elements from the SortedList?
- Clear: To retrieve the key/value pairs in the SortedList, use a foreach loop.
- Remove: This function removes the element with the specified key from a SortedList object.
- RemoveAt: A SortedList object's element at the supplied index is removed using this function.
Example:
Program:
The CODE is as follows:
// C# program to illustrate how to
// remove key/value pairs from
// the sortedlist
using System;
using System.Collections;
class Preethi {
// Main Method
static public void Main()
{
// Creating a sortedlist
// Using SortedList class
SortedList my_slist = new SortedList();
// Adding key/value pairs in SortedList
// Using Add() method
my_slist.Add(1.02, "This");
my_slist.Add(1.07, "Is");
my_slist.Add(1.04, "SortedList");
my_slist.Add(1.01, "Tutorial");
foreach(DictionaryEntry pair in my_slist)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
Console.WriteLine();
// Remove value having 1.07 key
// Using Remove() method
my_slist.Remove(1.07);
// After Remove() method
foreach(DictionaryEntry pair in my_slist)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
Console.WriteLine();
// Remove element at index 2
// Using RemoveAt() method
my_slist.RemoveAt(2);
// After RemoveAt() method
foreach(DictionaryEntry pair in my_slist)
{
Console.WriteLine("{0} and {1}",
pair.Key, pair.Value);
}
Console.WriteLine();
// Remove all key/value pairs
// Using Clear method
my_slist.Clear();
Console.WriteLine("The total number of key/value pairs"+
" present in my_slist:{0}", my_slist.Count);
}
}
OUTPUT:
The Output for the following program will be:
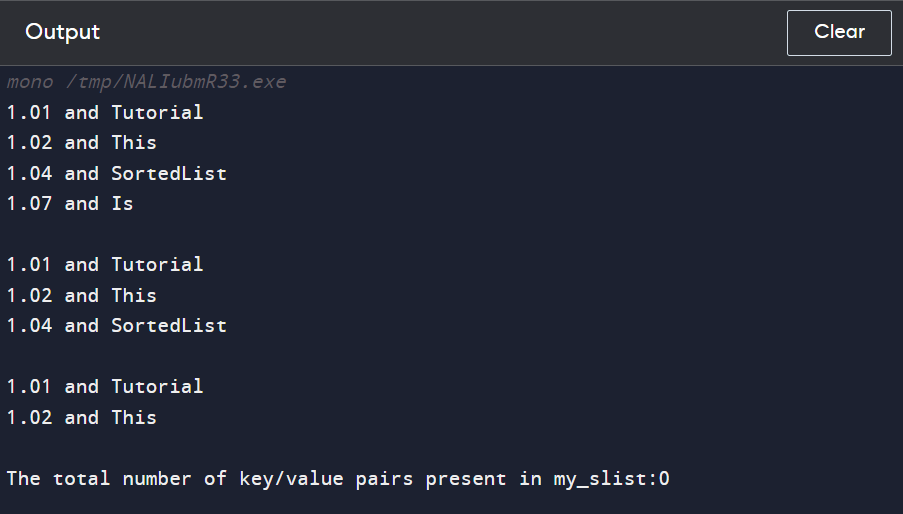
How to check the availability of key/value pair in SortedList?
You can use the following methods to determine whether the specified pair is present in the SortedList:
- Contains: Use this function to determine whether a SortedList object has a particular key.
- ContainsKey: Use this method to determine whether a SortedList object has a particular key.
- ContainsValue: Use this method to determine whether a SortedList object has a particular value.
Example:
Program:
The CODE is as follows:
// C# program to illustrate how to
// check the given key or value
// present in the sortedlist or not
using System;
using System.Collections;
class Preethi {
// Main Method
static public void Main()
{
// Creating a sortedlist
// Using SortedList class
SortedList my_slist = new SortedList();
// Adding key/value pairs in
// SortedList using Add() method
my_slist.Add(1.02, "This");
my_slist.Add(1.07, "Is");
my_slist.Add(1.04, "SortedList");
my_slist.Add(1.01, "Tutorial");
// Using Contains() method to check
// the specified key is present or not
if (my_slist.Contains(1.02) == true)
{
Console.WriteLine("Key is found...!!");
}
else
{
Console.WriteLine("Key is not found...!!");
}
// Using ContainsKey() method to check
// the specified key is present or not
if (my_slist.ContainsKey(1.03) == true)
{
Console.WriteLine("Key is found...!!");
}
else
{
Console.WriteLine("Key is not found...!!");
}
// Using ContainsValue() method to check
// the specified value is present or not
if (my_slist.ContainsValue("Is") == true)
{
Console.WriteLine("Value is found...!!");
}
else
{
Console.WriteLine("Value is not found...!!");
}
}
}
OUTPUT:
The Output for the following program will be:
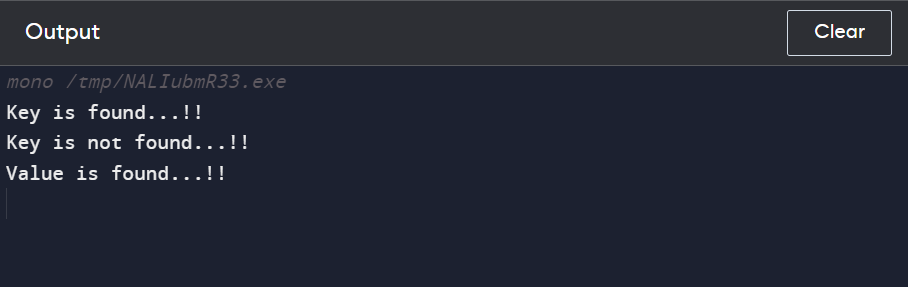
Key/value pairs with sorted keys comprise a non-generic collection called a sorted list. For example:
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program
{
public static void Main()
{
// create a SortedList
SortedList myList = new SortedList();
myList.Add(2, "Python");
myList.Add(1, "Java");
myList.Add(3, "C");
// iterate through myList
for (int i = 0; i < myList.Count; i++)
{
Console.WriteLine("{0} : {1} ", myList.GetKey(i),
myList.GetByIndex(i));
}
}
}
OUTPUT:
The Output for the following program will be:
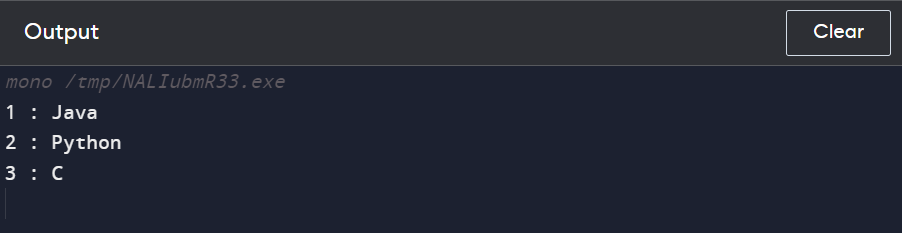
Basic Operations on a Sorted List:
On a SortedList, various C# operations are possible. In this tutorial, we'll examine a few frequently used SortedList operations:
1. Add Elements
2. Access Elements
3. Change Elements
4. Remove Elements
1. Add Elements in SortedList: The Add() function in C# allows us to add elements to a SortedList. For example:
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program
{
public static void Main()
{
// create a SortedList and add items
SortedList person = new SortedList();
person.Add(2, 45);
person.Add(1, "Jack");
person.Add(3, "Florida");
}
}
OUTPUT:
The Output for the following program will be:

A SortedList named person was constructed in the example above.
2. Access the SortedList: The SortedList's keys can be used to access its elements. For example,
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program
{
public static void Main()
{
SortedList myList = new SortedList();
myList.Add(2, "Python");
myList.Add(1, "Java");
myList.Add(3, "C");
// access the element whose key is 2
Console.WriteLine("Element whose key is 2: " + myList[2]);
// access the element whose key is 1
Console.WriteLine("Element whose key is 1: " + myList[1]);
}
}
OUTPUT:
The Output for the following program will be:
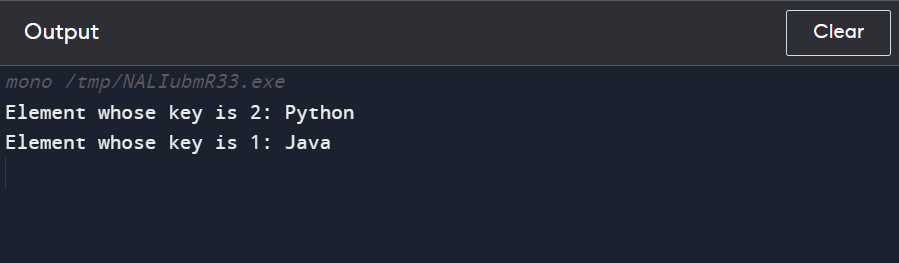
We have used the items' keys to access them in the example above:
Accesses the entry whose key is 2 in myList[2].
Accesses the element whose key is 1 in myList[1].
NOTE: When accessing, if we pass a key that doesn't exist, the compiler throws an error.
3. Iterate through SortedList: In C#, a for loop can be used to iterate through each entry of a SortedList repeatedly.
For example,
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program
{
public static void Main()
{
SortedList myList = new SortedList();
myList.Add(2, "BMW");
myList.Add(1, 96);
myList.Add(3, "Pizza");
// iterate through myList
for (int i = 0; i < myList.Count; i++)
{
Console.WriteLine("{0} : {1} ", myList.GetKey(i),
myList.GetByIndex(i));
}
}
}
OUTPUT:
The Output for the following program will be:
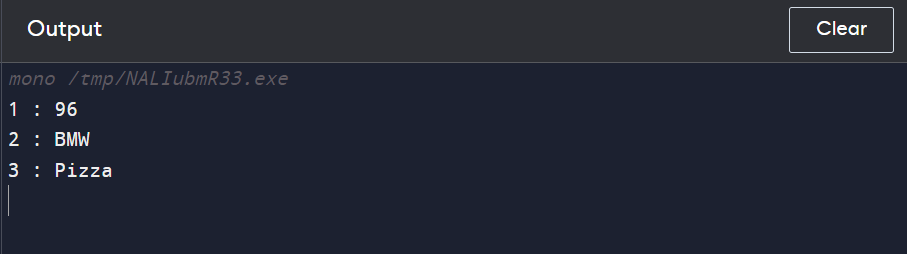
In the example above, we iterated through myList using a for loop.
We employ the GetKey() and GetByIndex() methods to retrieve the SortedList's keys and values.
The keys are sorted in the Output because the SortedList puts the entries in ascending order.
Note: The Count property counts the List's total number of elements.
4. Remove SortedListed Elements: There are two ways to remove entries from a SortedList:
Remove(key) eliminates the element using the given key
Removes the element based on the supplied index using RemoveAt(index).
Here are some examples using both methods:
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program {
public static void Main() {
SortedList myList = new SortedList();
myList.Add(2, "Evermore");
myList.Add(1, "Reputation");
myList.Add(3, "Folklore");
// remove element whose key is 1 i.e "Reputation"
myList.Remove(1);
// iterating through the modified SortedList
for (int i =0; i< myList.Count; i++) {
Console.WriteLine("{0} : {1} ", myList.GetKey(i),
myList.GetByIndex(i));
}
}
}
OUTPUT:
The Output for the following program will be:
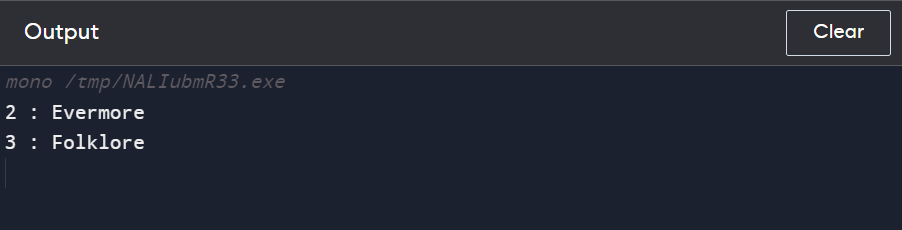
The element with key 1 has been eliminated from the example above.
Here is myList."Reputation" is taken away from myList by Remove(1). A changed list is produced as a result of iterating through myList.
Example: RemoveAt() Method:
Program:
The CODE is as follows:
using System;
using System.Collections;
class Program {
public static void Main() {
SortedList myList = new SortedList();
myList.Add(2, "Evermore");
myList.Add(1, "Reputation");
myList.Add(3, "Folklore");
// remove the element which is present in index 1 i.e "Evermore"
myList.RemoveAt(1);
// iterating through the modified SortedList
for (int i =0; i< myList.Count; i++) {
Console.WriteLine("{0} : {1} ", myList.GetKey(i),
myList.GetByIndex(i));
}
}
}
OUTPUT:
The Output for the following program will be:
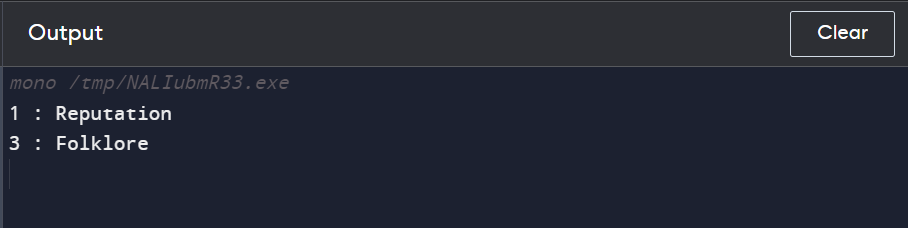
Example:
Program:
The CODE is as follows:
using System;
using System.Collections;
namespace CollectionsApplication {
class Program {
static void Main(string[] args) {
SortedList sl = new SortedList();
sl.Add("001", "Zara Ali");
sl.Add("002", "Abida Rehman");
sl.Add("003", "Joe Holzner");
sl.Add("004", "Mausam Benazir Nur");
sl.Add("005", "M. Amlan");
sl.Add("006", "M. Arif");
sl.Add("007", "Ritesh Saikia");
if (sl.ContainsValue("Nuha Ali")) {
Console.WriteLine("This student name is already in the list");
} else {
sl.Add("008", "Nuha Ali");
}
// get a collection of the keys.
ICollection key = sl.Keys;
foreach (string k in key) {
Console.WriteLine(k + ": " + sl[k]);
}
}
}
}
OUTPUT:
The Output for the following program will be:
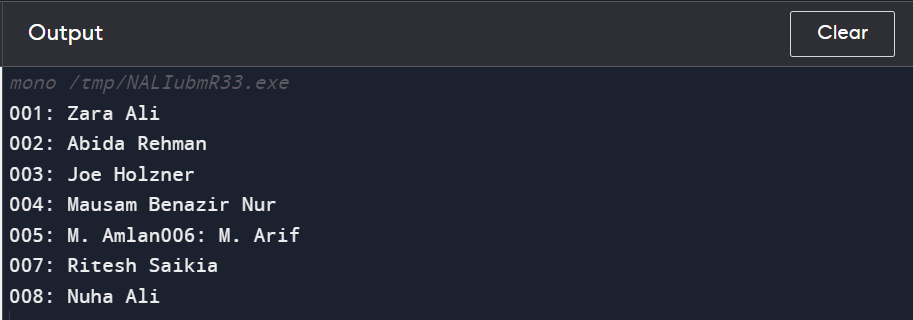
SortedList Characteristics:
- Immediately sorts components after their addition. Sorts object keys based on IComparer<T> and primitive type keys in ascending order.
- Belongs to System.Collection.Generic namespace.
- A key cannot be null and must be unique.
- A value can be obtained by entering the associated key in the indexer mySortedList[key].
- Consists of components of the type KeyValuePair<TKey, TValue>.
- Compared to SortedDictionary<TKey, TValue>, it consumes less RAM.
- It performs data retrieval more quickly than SortedDictionary<TKey, TValue>, which performs key-value pair insertion and removal more quickly.
Conclusion:
In Conclusion, the C# SortedList class is a compelling collection that enables you to hold key-value pairs in a sorted order based on the keys. It offers effective operations for adding, accessing, and removing elements by combining the capability of a dictionary and a sorted array. You can interact with sorted data in your C# programs with the SortedList.
The SortedList class provides several properties and methods for modifying and querying the collection. By defining the types for the keys and values, you can construct a SortedList. Then, you may add entries using the Add method. The indexer notation is used to access elements, and each loop can traverse through the elements sequentially in ascending order.
The SortedList class also includes methods to get the collection's element count, determine whether a key exists, and remove elements from the List. You may also get collections of keys and values independently using the Keys and Values attributes.
You may easily manage sorted data and perform operations using the SortedList class. The SortedList class in C# helps complete such tasks, whether you need to maintain a sorted collection of items.