How to handle null exception in C#
A NullReferenceException in C# is raised when we attempt to access a variable whose value is null or has not been set at all. It's crucial to understand how to prevent this exception in the first place because it can be simple to unintentionally cause it. This post will examine some typical reasons for NullReferenceException issues as well as solutions. Additionally, we'll go over how to stop NullReferenceException issues from occurring in the first place.
Null reference in C#
A pointer that does not point to a legitimate memory address or object is called a null pointer or null reference. This issue has existed for as long as programmers have been writing programs.
A null value is a unique value that indicates that a valid value is not available. When a null value is applied to an object, all fields and variables within the object are reset to null or empty. You must manage null values in your application's code to prevent null reference exceptions and unpredictable runtime behaviour.
A NullReferenceException: What causes it?
In C#, references to objects are stored in variables of reference types. The null value is used to indicate that the reference does not point to an object. It's also important to remember that reference-type expressions have null as their default value.
When you attempt to dereference a null reference, an exception of the NullReferenceException type is raised. Below is a list of some examples of these operations.
Example:
An example of the code that raises a NullReferenceException is provided below:
using System;
using System.Collections.Generic;
public class Example
{
public static void Main()
{
IList<string> students = null;
ShowStudents(students);
}
public static void ShowStudents(IList<string> students)
{
foreach (var student in students)
{
Console.WriteLine(student);
}
}
}
Because the students list in the example above is null, the ShowStudents() function will throw a NullReferenceException when accessing the cities list using a foreach loop. The same exception will be raised by the ShowStudents() function if its caller passes in a null IList parameter.
Output:
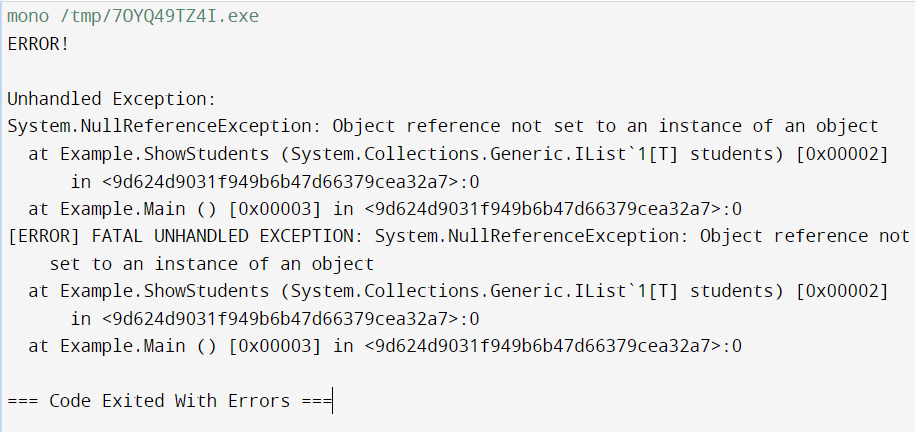
Exclude the case of null references dereference to prevent exceptions of the NullReferenceException class. Use these steps to accomplish this:
- Determine the source of the null reference and how it enters an expression;
- modify the application's logic to prevent null reference access.
How to handle NullReferenceException
Before accessing the reference type parameters, make sure they are not null to avoid raising the NullReferenceException exception.
Example 1: Use an if condition to determine if an object has a null value or not, as demonstrated below:
using System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
IList<string> students = null;
ShowStudents(students);
}
public static void ShowStudents(IList<string> students)
{
if (students == null) //before accessing check for null
{
Console.WriteLine("No Students");
return;
}
foreach (var student in students)
{
Console.WriteLine(student);
}
}
}
If(students == null) in the example above determines whether or not the students object is null. Display the relevant message and exit the function if it is null.
Output:
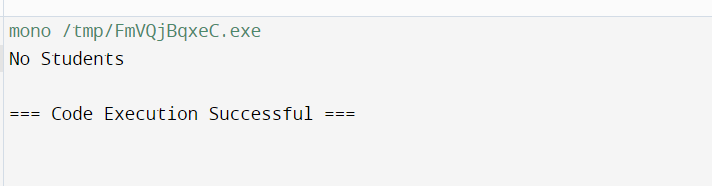
Example 2: As seen below, utilize the null conditional operator “?” in.NET 5:
using System;
public class Program
{
public static void Main()
{
Person prsn = null;
Display(prsn);
prsn = new Person(){ Name = "Puja Murthy", MobileNumber = "9876543210"};
Display(prsn);
}
public static void Display(Person prsn)
{
Console.WriteLine("Person Details:");
Console.WriteLine(prsn?.Name);
Console.WriteLine(prsn?.MobileNumber);
}
}
public class Person
{
public int sno { get; set; }
public string Name { get; set; }
public string MobileNumber { get; set; }
}
In the illustration above, prsn?.The name is similar to if(prsn!= null) std.Name. The prsn?.Name only accesses the Name property after determining that prsn is not null.
Output:
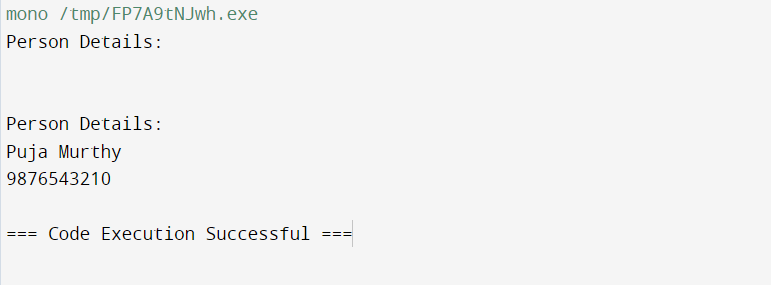
Example 3: Use the Null-Coalescing operator "??" in.NET 4.x and later versions. To avoid an exception, as demonstrated below:
using System;
using System.Collections.Generic;
public class Example
{
public static void Main()
{
IList<string> students = null;
ShowStudents(students);
}
public static void ShowStudents(IList<string> students)
{
foreach (var student in students?? new List<string>())
{
Console.WriteLine(student);
}
}
}
The null coalescing operator in the example above,??, determines whether an object is null or not and creates an object if it is. If a student in the foreach loop is null, the students?? new List() function generates a new list object. As a result, no NullReferenceException will be triggered.
Output:
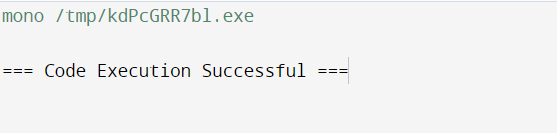