TouchableOpacity in React Native
Touchable opacity is the most suited example if you want to make views respond to proper touch. If you want to control the opacity then it can be done by wrapping the children into it. Elements are called children of the App.
Props of touchable opacity :
activeOpacity: It determines the level of opacity that should be present when touch is active. Its default value is 0.2
hasTvpreffredFocus: It is of boolean type taken only true or false.
nextFocusDown: it accepts only numbers as arguments.
nextFocusNumbers: It also accepts only numbers as arguments.
Some same props named nextFocusRight,nextFocusLeft,nextFocusUp all of
them accepts only neumeric value.
App.js code:
import React, { Component, } from 'react'
import {
StyleSheet,
View,
Text,
TouchableOpacity,
} from 'react-native'
export default TouchableOpacityExample = (props) => {
return (
<View style = {styles.container}>
<TouchableOpacity style={{backgroundcolor:'pink'}}>
<Text style = {styles.button}>
example
</Text>
</TouchableOpacity>
</View>
)
}
const styles = StyleSheet.create ({
container: {
flex: 1,
backgroundcolor:'pink',
borderWidth:1,
alignItems: 'center',
justifyContent: 'center',
},
button: {
borderWidth: 1,
padding: 25,
justifyContent: 'center',
backgroundcolor:'red',
fontsize:25
}
})
Output :
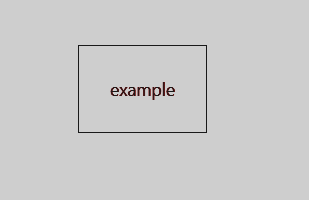
Explanation:
We have imported StyleSheet, View, Text, and TouchableOpacity because we will be using them throughout the app.
<View style = {styles.container}>
<TouchableOpacity style={{backgroundcolor:'pink'}}>
<Text style = {styles.button}>
example
</Text>
</TouchableOpacity>
</View>
We have declared our button and given it the name “ Example”, you can see within the Text we have written “example” and it will appear inside the box in output which you can see in the output.
The stylesheet is for styling purposes. Through container, we have styled the whole rendered app but the button is specific for touchable opacity and you can see in touchable opacity code within styling we have mentioned {styles.button} so it inherits styles from the button.
Lets see one more example-
Example - 2
App.js code:
import React, { Component } from 'react'
import {
StyleSheet,
TouchableOpacity,
Text,
View,
} from 'react-native'
export default class App extends Component {
constructor(props) {
super(props)
this.state = { count: 10 }
}
onPress = () => {
this.setState({
count: this.state.count+10
})
}
render() {
return (
<View style={styles.container}>
<TouchableOpacity
style={styles.button}
onPress={this.onPress}
>
<Text> click here </Text>
</TouchableOpacity>
<View style={[styles.countContainer]}>
<Text style={[styles.countText]}>
{ this.state.count !== 0 ? this.state.count: 100}
</Text>
</View>
</View>
)
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
backgroundColor:'pink',
paddingHorizontal: 10
},
button: {
padding: 25,
alignItems: 'center',
backgroundColor: '#DDDDDD',
alignItems:'center',
justifyContent:'center',
borderWidth:1,
padding: 10
},
countContainer: {
alignItems: 'center',
padding: 15,
color:'red'
},
countText: {
color: 'red',
fontsize:25
}
})
Output
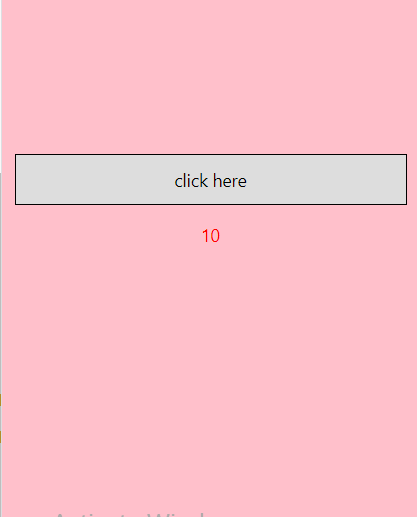
Explanation:
import React, { Component } from 'react'
import {
StyleSheet,
TouchableOpacity,
Text,
View,
} from 'react-native'
We are importing components as per our need such as stylesheet,touchableopacity,text and view.
this.state = { count: 10 }
This sets the default value of the count, which is 10. So initial value will of the count be 10 and it will increase as per our command.
onPress = () => {
this.setState({
count: this.state.count+10
})
}
This is the responsive part which decides what will happen if the button is clicked. So as per code, it will be incremented by 10.
Ex- default value is 10. Once clicked, it will be 20, the next 30, etc.
then we do the declaration of a button. Through touchable opacity, we are constructing a button. The text defines the title of the button and styling is done using a stylesheet. The code within the stylesheet is for styling each component such as container, count text, count button, etc.