Redux in React Native
It is a really amazing library of javascript, react, and react-native. It is very popular in react native domain. Every react and react native developer must know the redux it is compulsory in order to become a proficient react native and react developer. It is used for state management of an application. An application consists of huge data, so redux will simplify managing it. Using the conventional methods for managing the data will make code complex and bulky, so a redux library is made to solve this problem. It is mainly used in the universal app. The properties are stored in one object rather than creating multiple objects and storing them individually. If you want to change the property, you cannot do this directly. You have to make a new object and then recalculate the whole application, and then the application will be updated with the newly created object.
Why redux? Or Drawbacks of conventional method :
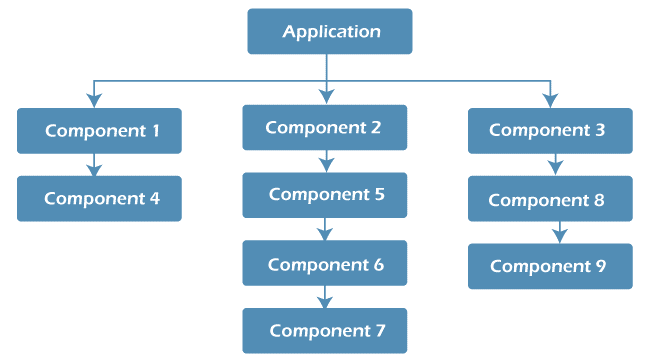
Suppose you want to change the state of component seven, so through the conventional method, the possible way to do this is, first of all, you need to make all the existing states available in App.js. The selected state will first pass to component 2, and .then the passing sequence will be component 5 to component 6 to component 7(our final state received).this is a very lengthy process as we only want to change the state of one component. Here we have to go through 3 different components to execute our plan, and these three components have nothing to do with this. Suppose if there were 40 components between app.js and component 7, then we would have to travel through 40 components, and then we would be able to change the state of component 7, so this is the main drawback of the conventional method. Now the question arises of how redux helps to do the same task in an optimized manner. In the next part working of redux is explained. There you can learn how redux can do the same task but in an easy way.
Working of redux simplified
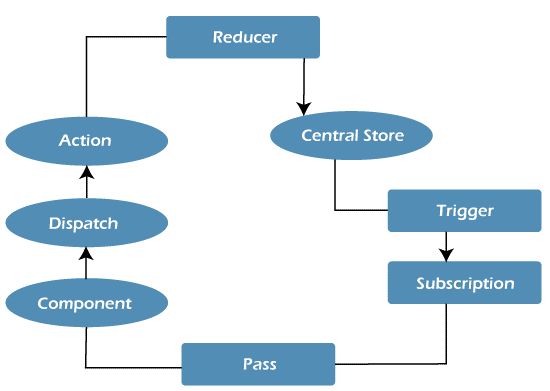
Here we have 8 different parts, all of which have their own responsibility or work to do.
Action: The component dispatches the action. It delivers the message about what is going on in the application. Only action contains information about the incident(what has happened), and it also contains the payload as well as some parts of the action that also tell what action is needed to perform. It is sometimes referred to as type property. When the action is dispatched, all available states will be triggered.
Syntax of action :
Const action:{
Type:” “,
Payload: “”,
}
Here you can see two constraints of action. The first is the type and the second is the payload. The payload contains extra information about the event, which may or may not be included during the action declaration. Including type while declaring an action is compulsory and should be of type string.
Reducer: It is one of the pure functions of redux. It performs the main function of state-changing. We can write logic here. It will accept action from the previous state of the application, as shown in the diagram. After receiving the previous state, it executes and checks the logic, recalculates the new state, and returns a new object. Redux helps to know how to respond to a given condition.
The reducer cannot perform the following actions:
- We cannot perform the Manipulation of function arguments of redux.
- We cannot call any impure functions using redux.
- It cannot call API(Application Programming Interface) as well as a subroutine.
Syntax of reducer:
(state,action)=> newState
It takes state and action as arguments and returns a new state, or we can say it returns a new object. Direct modification of state is prohibited, so make a copy of the new state. New states are still not updated in the main App.js.
What is a Pure function?
The purity of any function is judged based on its return type. Suppose it returns a value determined by its given input. The pure function always returns the same value for the same input. It does not show any kind of side effects. If any function's return type is different from its given input value, then it is said to be an impure or non-pure function and always shows side effects.
Example :
x=4;
y=5;
Return x*y;
Here we know the output will be 20, and its type will be an integer as x and y both are of integer type, so it returns an integer the same as the input. So this mechanism is the same as a pure function.
Rest functions are store, trigger, subscription, and pass. once the new object is recalculated, it is passed to store, and then final modification of the state is done by triggering the object and subscription; lastly, the new state is passed to the component, and hence the state of the component is changed.
Installing redux tool kit -
(just run any of the following commands in your terminal)
- npm, install @reduxjs/toolkit.
or
- yarn install @reduxjs/toolkit.
Making react-redux app:
(just run any of the following commands in your terminal)
- npx create-react-app my-app --template redux
Making react-redux app with typescript:
- npx create-react-app my-app --template redux-typescript
Installing core redux:
(just run any of the following commands in your terminal)
- Npm install redux
or
- Yarn install redux
Example of redux :
Create two files named mycompo.js and list_1.js.
Mycompo.js:
export const GET_REPOS = 'my-awesome-app/repos/LOAD';
export const GET_REPOS_SUCCESS = 'my-awesome-app/repos/LOAD_SUCCESS';
export const GET_REPOS_FAIL = 'my-awesome-app/repos/LOAD';
export const GET_REPOS_SUCCESSful = 'hello';
export const first='fine';
export const second='fine';
export const third='fine';
export const fourth='fine';
export const fifth='fine';
export const sixth='fine';
export default function reducer(state = { repos: [] }, action) {
switch (action.type) {
case GET_REPOS:
return { ...state, loading: true };
case GET_REPOS_SUCCESS:
return { ...state, loading: false
, repos: action.payload.data };
case GET_REPOS_FAIL:
return {
...state,
loading: false,
error: 'Error while fetching repositories of this folder'
};
case GET_REPOS_SUCCESSful:
{
};
case first:
{
};
default:
return state;
}
}
export function listRepos(user) {
return {
type: GET_REPOS,
payload: {
request: {
url: `/users/${user}/repos`
}
}
};
}
List.js
import React, { Component } from 'react';
import { View
, Text
, FlatList
, StyleSheet } from 'react-native';
import { connect } from 'react-redux';
import { listRepos } from './mycompo';
class Repo_List extends Component {
componentDidMount() {
this.props.listRepos('relferreira');
}
renderItem
= ({ item }) => (
<View style={styles.item}>
<Text>{item.name}</Text>
</View>
);
render(){
const{ repos } = this.props;
return(
<FlatList
styles={styles.container}
data={repos}
renderItem={this.renderItem}
/>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
color:'pink',
padding: 25,
},
item: {
borderBottomWidth: 10,
borderBottomColor: 'pink'
}
});
const mapState_To_Props = state =>{
let stored_Repositories
=
state.repos.map(repo =>
({ key: repo.id, ...repo }));
return {
repos: stored_Repositories
};
};
const map = {
listRepos
};
export default connect(mapState_To_Props, map)(Repo_List);
App.js:
import React, { Component } from 'react';
import { StyleSheet
, View
, Text } from 'react-native';
import { createStore, applyMiddleware } from 'redux';
import { Provider, connect } from 'react-redux'; import axios from 'axios';
import axiosMiddleware from 'redux-axios-middleware';
import reducer from './mycompo';
import RepoList from './List_1';
const client = axios.create({
baseURL: 'https://api.github.com',
responseType: 'json'
});
const store_first = createStore(reducer,
applyMiddleware(axiosMiddleware(client)));
export default class App extends Component {
render(){
return(
<Provider store={store_first}>
<View style={styles.container1}>
<RepoList />
</View>
</Provider>
);
}
}
const styles = StyleSheet.create({
container1: {
flex: 10,
backgroundColor: 'white',
marginTop: 25,
borderwidth:'10'
}
welcome: {
flex:1,
fontSize: 25,
textAlign: 'center',
margin: 15
}
}
)
item: {
flex:1,
fontSize: 25,
textAlign: 'center',
margin: 15
}
}
)
});
Output:
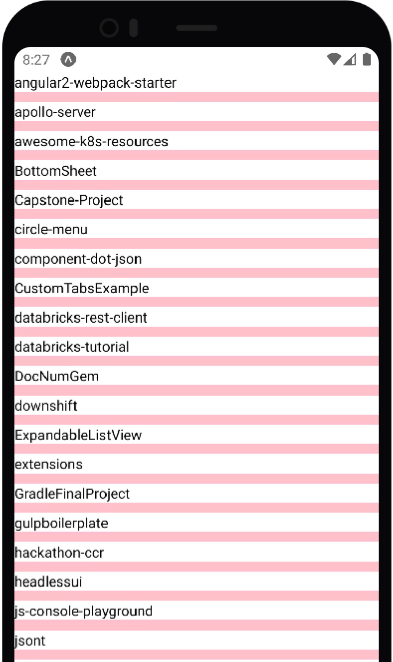
Explanation:
Let us understand mycompo.js first -
export const GET_REPOS_SUCCESS = 'my-awesome-app/repos/LOAD_SUCCESS';
export const GET_REPOS_FAIL = 'my-awesome-app/repos/LOAD_fail';
Here we had multiple repositories, but we will be focusing on these two as they are associated with success and failure. Rest are just exported, and no other methods are included. Here we have put the repository in one constant, and we cannot copy the whole repository at once. We have just put the path so that the constant can access it. The case of success and failure is given below.
case GET_REPOS_SUCCESS:
return { ...state, loading: false
, repos: action.payload.data };
The success function is where we are supposed to return payload data directly. Here loading is made false as we will access the repository.
case GET_REPOS_FAIL:
return {
...state,
loading: false,
error: 'Error while fetching repositories of this folder.'
};
, repos: action.payload.data };
The failure function is where we are supposed to return an error message. Here, loading is made false as we access the repository, but an error message will be displayed.
Then we have declared the argument and given type as well as payload(we have already discussed these terms earlier ), and lastly, the state is exported to the App.js function.
List_1.js code explanation:
Firstly we have exported all necessary component such as text,view and stylesheet etc. then we have included redux using-import { connect } from 'react-redux'; the list figure of output is constructed in this file and then attached to App,js .
<FlatList
styles={styles.container}
data={repos}
renderItem={this.renderItem}
/>
We have used function to render or access the available files, and then they are put into constant, which is used in the flat list component to construct a list of available files. Each item has its own id. This is the terminology behind the available list of files. Lastly, we have used a stylesheet to make the available list neat and clean in appearance.
App.js code explanation:
All the necessary components are exported first. All the necessary elements of redux are exported to our app, such as create the store,applyMiddleware, provider connect, etc. we have defined one API in client constant, which will be rendering all the files. The data fetched by API is in JSON format. JSON stands for javascript object notation. Which is here used for giving title to the flat list. you can see names such as apollo server, bottom sheet, circle menu, etc. this is the exported JSON from API and used in the flat list. Rest we have used styling to style some components.