React Native Sound
It is a great way to connect to the user. Just a tiny "ting" of any email received, or some sound for deleting a file or sound for sending a message can create an impact and a better user experience for our react-native app. react-native sound can help us to engage with users. Sound often makes using the app easy by just some sound, we can understand what has happened, and it also helps us to differentiate between different tasks without looking at the screen.
React native has a sound component to control all sound-related issues. It is a powerful tool that helps us manage sound properties. It is a little advanced topic, so firstly, you must be familiar with the basics of react native, its components, and some methods. This component has a method for sound playing, which is applicable for Android, IOS, and Windows. We can add the sound file from various resources. It is easy to add them to our app, but you need to have some basic understanding of react-native for a smooth learning experience. The instance can be controlled by its predefined methods. One drawback or concern is that the documentation of react-native sound is the alpha type which means that it can contain bugs, but it is not the barrier to using it. Various developers use it. It is used in many apps despite its alpha-type documentation. It is a powerful component. We will be doing practical of it and adding sound as well as controlling it, but before that, we need to know some basic theory of react-native sound which is explained below.
What is react native sound?
Nowadays, the react-native sound is used in various famous applications. It is a module that allows us to add sound to our app from various resources, which can be controlled using some methods. Its property makes it more of a class than a class of components. It is one of the most used libraries and methods worldwide for adding sound to our react native app. The sound makes the user experience awesome it makes the app alive. You may have observed it while using any app. its alpha-type documentation is a concern, but still, it works fine and smooth. Nowadays,
setting the environment for react-native sound( Installing the necessary packages) :
Sounds are often unavailable remotely. You need to have those files available (it should be an in-app package rather than your external storage or any remote server).
Run the following command in your terminal -
Npm add install react-native-sound
Or
Yarn add install react-sound-native
If you are using IOS, then navigate to the directory. You need to install pods. Pods are required for adding react-native sound. Just call-pod install. This will add all the required pod for your react native sound.
Adding sound to our react native app :
Firstly we need to import the sound from our local directory. Only then we can add those to our app.
So now, let's do the practical implementation of the react-native sound. Firstly paste any sound file or your desired file to the apps directory and do not forget to see the path for it, and then import it from the existing folder for our app. The import will be done in the necessary part of the app. It can be the main app.js file, or you can create any separate file. It's your choice or requirement, but if the availability of a music file inside the apps directory is mandatory, then you can add it to the app as per your requirement. Then we will be learning the code part by part.
App.js code:
import {View, StyleSheet, TouchableOpacity} from 'react-native';
import React, {useEffect} from 'react';
import tings from './assets/ting.mp3';
var Sound1 = require('react-native-sound');
Sound1.setCategory('Playback');
var ding = new Sound1('ting.mp3', Sound1.MAIN_BUNDLE, (error) => {
if (error) {
console.log('failed to load the available sound', error);
return;
console.log('seconds duration : ' + ting.getDuration() + 'the total number of channels: ' + ding.getNumberOfChannels());
});
const App = () => {
useEffect(() => {
ting.setVolume(2);
return () => {
ting.release();
};
}, []);
const playPause = () => {
ting.play(success => {
if (success) {
console.log('so! we successfully finished playing the aviavlble sound');
} else {
console.log('there are some audio decoding error so we failed to play the sound');
}
});
};
return (
<View style={styles.container}>
<TouchableOpacity style={styles.playBtn} onPress={playPause}>
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: 'white ',
},
playBtn: {
padding: 20,
backgroundColor: "black",
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
play_Btn: {
padding: 20,
backgroundColor: "black",
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
});
export default App;
Output:
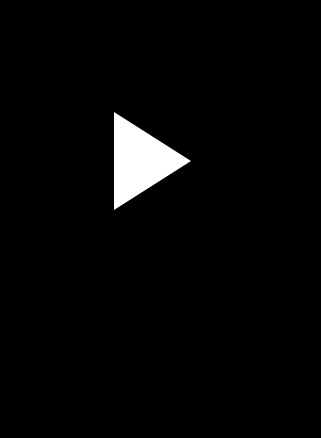
You can see we are getting one play button in output. If you click on it, the sound will start playing, and if the sound is not playing, it has some problems so letting the user know about the problem is an important factor, so we have attached one exception handling method too. when there is a problem in playing, then a message will be displayed on the screen, which is -
"There are some audio decoding errors, so we failed to play the sound', and if there is no problem."
If there is no problem, then it will simply play the given sound file, and a message will be displayed on the screen, which is -
“so! we successfully finished playing the available sound."
Explanation of code :
Firstly we have to import all the required files and our music file. We have to ensure that the required file is in the app, which means it should be in the app's directory. You can add it to an asset or any folder. You even can create a folder and then add the sound there, or the sound file can be present there individual you need the path for accessing the file. A path will be different for different directories. Suppose I want to import a music file inside my music folder, which is in the asset, so how can I access it? The following code can be used - import things from './assets/mymusic/ting.mp3.
And if the music is present individually so we can use - import tings from './ting.mp3 , you should be concerned with the extension here my file is of mp3 type that why I have used mp3 and if the file is of mp4 then we have to write mp4 in the place of mp3.
There can be two types of error. The first one is the app cannot access the file, so if that happens. You will get a message which is - failed to load the available sound' error. We have tried to handle both the exceptions. Let us understand the code -
if (error) {
console. log('failed to load the available sound', error);
return;
So we are trying to load the music file within the var variable through the main bundle. If we cannot do so, then there must be some error, and you can see within the if statement we have written error and as there is an error so it will be true, and then the message will be printed, and it will return. Then the developer can check the existing file and try to solve the issue, and after solving the bug, the sound can be played successfully. Let us look at the second error where the sound is accessing correctly, but there is trouble playing it.
ting.play(success => {
if (success) {
console.log('so! we successfully finished playing the aviavlble sound');
} else {
console.log('there are some audio decoding error so we failed to play the sound');
}
});
Here if the sound is played successfully, then so! We successfully finished playing the available sound' this message will be displayed, and if there is a bus, then if(success) will become false as there is nothing inside the success, so if the statement is not executed, then the control will move to else part
. You can see the message inside another part: ' there are some audio decoding errors, so we failed to play the sound', which will be displayed. Then the developer can check the existing file and try to solve the issue, and after solving the bug, the sound can be played successfully. Let us look at the second error where the sound is accessing correctly, but there is trouble playing it.
<TouchableOpacity style={styles.playBtn} onPress={playPause}>
We have created a button and there is onpress property which calls playpause when pressed, we know onPress defines the response of the button when it will be pressed and then two things can happen either the music will be played or the error message will be displayed. The button is important because it makes the UI(user interface ) clean and efficient.
Rest code is for the styling part we have used the stylesheet and styled all components as per our needs and requirement.
We can add sound to our app using a remote server, just try to understand the code below -
App.js code
import {React} from 'react';
import {View, TouchableOpacity, StyleSheet} from 'react-native';
import{useState,useEffect, } from 'react';
var Sound = require('react-native-sound');
Sound.setCategory('Playback');
var mysound = new Sound(
'your_url_for_music',
null,
error => {
if (error) {
console.log('failed to load the avaiavle sound', error);
return;
}
console.log(
'seconds durtaion of file: ' +
mysound.getDuration() +
'quantity of channels: ' +
mysound.getNumberOfChannels(),
);
},
);
const App = () => {
const [playing, setPlaying] = useState();
useEffect(() => {
mysound.setVolume(4);
return () => {
mysound.release();
};
}, []);
const play_and_Pause = () => {
if ( mysound.isPlaying()) {
mysound.pause();
setPlaying(false);
} else {
setPlaying(true);
mysound.play(success => {
if (success) {
setPlaying(false);
console.log('successfully finished playing');
} else {
setPlaying(false);
console.log('playback failed due to audio decoding errors');
}
});
}
};
return (
<View style={styles.container}>
<TouchableOpacity style={styles.play_Btn} onPress={play_and_Pause}>
</TouchableOpacity>
</View>
);
};
const styles = StyleSheet.create({
container: {
justifyContent: 'center',
flex: 1,
backgroundColor: '#000',
alignItems: 'center',
},
play_Btn: {
padding: 20,
justifyContent: 'center',
flex: 1,
},
});
export default App;
Output :
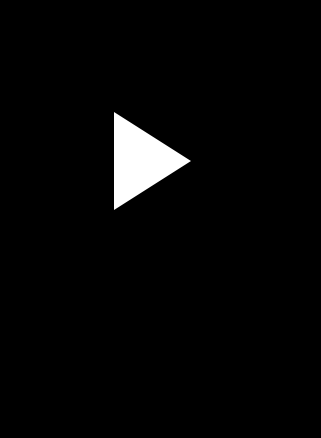
Here we can see a play button it will be used to play and pause the music. If the audio file is not accessible, then you will not be able to see the button. As you can see, here is a button that means the audio is accessible, and we have attached it to our app through the remote server.
Explanation of code :
Firstly we have to import all the required files. Let's see how the music is attached to the app-
var my sound = new Sound(
'your_url_for_music',
Here you have to replace the 'your_url_for_music' with the music's URL for the demo. It can be like - "hellosound.mp3/my_music.com".rest we have added exception handling here, which will print the respective message if any bug is detected so that developer can work accordingly and update the code. The rest code is for the styling part. We have used the stylesheet and styled all components per our needs and requirements.