JavaScript Beautifier
JavaScript: What is it?
Web applications frequently use JavaScript, a high-level, dynamic, and interpreted programming language. Since its original creation, it has developed into a flexible language that can be used for both front-end and back-end development. It was intended to make websites more interactive. Because of its widespread use, JavaScript has become a necessary language for web developers and is now considered a must for all aspiring developers.
Overview of the JavaScript formatter
A formatter in JavaScript is used to decrease the number of whitespaces in the code, minimize the amount of data that has to be communicated and provide the user with an overall better code format. Offering appropriate indentation and modification makes the entire collection of codes seem beautiful. The JavaScript formatter allows the user to select the desired level of formatting to improve the code's readability and reusability, ranging from text to String to object to code. The JavaScript Formatter gives the code a standard.
JavaScript scripts may be edited, viewed, analyzed, beautified, and formatted with this free online tool. It's a quick and easy method to make JavaScript code seem nice and organized for collaborations.
Syntax
The following is the JavaScript syntax for using Formatter to format a string:
String.prototype.format = function () {
var a = this;
for (var b in arguments) {
a = a.replace("{" + b + "}", arguments[b])
}
return a
}
The values of the string assigned in the reserved variable are finally represented in the syntactic flow, which starts with the function being assigned to the string format, replaced with the working variable, and then has the string formatted appropriately.
How does JavaScript formatter work?
- Although there is no set pattern or method for understanding a formatter in JavaScript, it is simple to grasp the Java formatter's flow if we can comprehend the formatter in a text-level manner.
- As previously stated, the JavaScript formatter operates based on the user and the formatting level that is chosen and processed at the text, string, object, and code levels. Thus, formatting is mostly used to enhance the code's visual appeal and user-friendliness.
- It is crucial to comprehend the designer's class demands while formatting content. The majority of JavaScript letters and text with words are already supported by built-in packages and APIs in Visual Studio Code, for example, so formatting in JavaScript also functions well if we integrate certain external plugins in the IDE.
- Text data in any format is represented by JavaScript's string type. Here, the formatter uses literals or string objects to generate the string after attempting to determine the character's position within the string and its length.
- When formatting a string object, be careful to check and pay attention to spaces and delimiters, since adding them might result in inappropriate indentation in a string generated as data.
- Using a prepared document, the string method may be used to alter text at the string level and carry out other formatting operations. An interesting internationalization API now includes complete support and inclusion for the format utilities Collator, NumberFormat, and DateTimeFormat.
- In contrast, the JavaScript formatter is crucial to web development as it enables precise formatting at precise times.
- When dealing with DateTimeFormatter in JavaScript, it is advised to utilize plugins and built-in standard libraries since datetime manipulation in JavaScript can be laborious owing to the rigid and complicated structure of the ISO standard.
- One pleasant perk for developers using JavaScript is the ability to use a formatter. By enhancing the code's visual attractiveness with suitable indentation, spacing, and alignment, this guarantees consistency.
JavaScript formatter examples
The following are some formatted examples of JavaScript:
Example 1
The formatter is shown by this program. HTML-based content may be formatted by the user by adding features, writing it in paragraph form, and utilizing tags for formatting. For instance, as can be seen in the output, the paragraph may be positioned to the right and the text font's color and type changed.
Code
<!DOCTYPE html>
<html>
<body>
<p> We may alter and modify this text.</p>
<p align= "Right"> This paragraph is aligned towards the Right.</p>
<p><i>The font of the article is changed.</b></i>
<p><font color="Red">The Font color formatted into Red color.</font></p>
</body>
</html>
Output
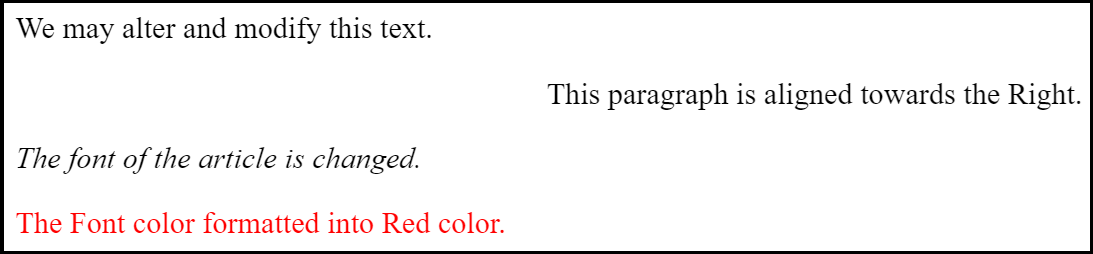
Example 2
This program shows how to change a text's string format in italics after clicking the submit button using JavaScript, as seen in the output.
Code
<!DOCTYPE html>
<html>
<body>
<p>Press on the submit button to display the string format in Italics</p>
<button onclick="Fun()">Submit</button>
<p id="main"></p>
<script>
function Fun() {
var one = "Welcome all..!";
var two = one.italics();
document.getElementById("main").innerHTML = two;
}
</script>
</body>
</html>
Output
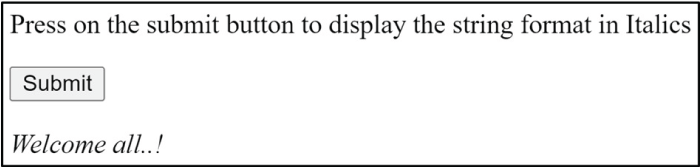
Example 3
Using JavaScript short Dates as a formatter to express the date in the "DD/MM/YYYY" syntax as displayed in the output, this program first shows the variable before creating a new date and assisting in the appropriate value transformation.
Code
<!DOCTYPE html>
<html>
<body>
<h1>Creation of JavaScript dates</h1>
<p id="main"></p>
<script>
var one = new Date("02/21/2002");
document.getElementById("main").innerHTML = one;
</script>
</body>
</html>
Output
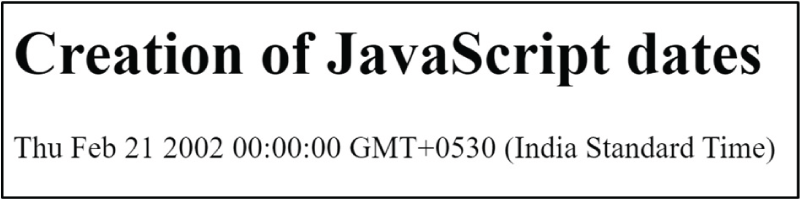
Example 4
By measuring the length and presenting it as shown in the output, this program illustrates the string formatter length and the associated attribute for that string object.
Code
<!DOCTYPE html>
<html>
<body>
<h1>Measuring length of JavaScript String formatter length</h1>
<p>Length of the String.</p>
<p id="main"></p>
<script>
var one = "Hello World!! Welcome to the tutorial";
var two = one.length;
document.getElementById("main").innerHTML = two;
</script>
</body>
</html>
Output
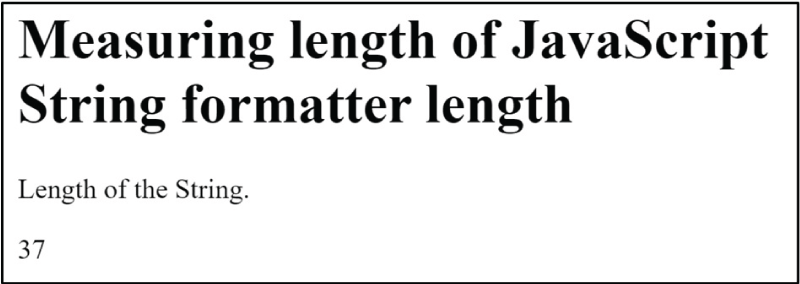
Example 5
This program shows how to format JavaScript data using ECMA Script in the defined property object and then manipulate all the values accordingly. It specifically instructs how to alter the property object's values as displayed in the output.
Code
<!DOCTYPE html>
<html>
<body>
<h2>Example of how to define object property</h2>
<p>Change in the Object's Property value.</p>
<p id="main"></p>
<script>
var stu = {
stu_Name: "ABC",
stu_id : "11",
stu_dept : "ECE"
};
Object.defineProperty(stu, "stu_dept", {value:"ECE"})
document.getElementById("main").innerHTML = stu.stu_Name;
</script>
</body>
</html>
Output
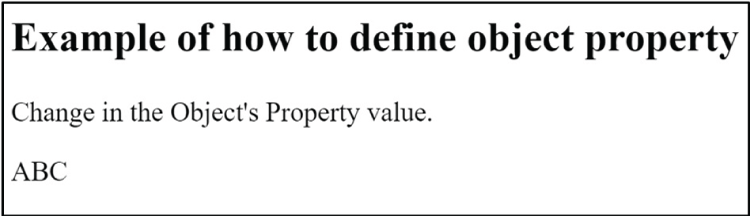
In summary
JavaScript Formatter is essential to web development because it allows programmers to create code more efficiently and elegantly by enabling them to alter and improve it. Additionally, because many developers collaborate, they have the advantage of maintaining consistency in the code during implementation with enhanced comprehension, ease of use, and ease of understanding.