How to Print a Page Using JavaScript
JavaScript is one of the most widely used programming languages globally, recognized for its portability, universality, and ease of understanding. It serves as a crucial scripting language for the internet, particularly in creating dynamic websites. JavaScript, a client-side scripting language used by all Modern browsers, enables web servers to access source code, send it over the World Wide Web to clients' computers, and then execute code directly in browsers, thereby facilitating the analysis of page behavior. Additionally, it is utilized for user event processing and checks. The present web page can be printed and stored in a format that can be transported thanks to Javascript.
This post will go over how to print a web page in Javascript and explain its implementation using an example.
Using Window print() Method
The "window.print()" method in JavaScript allows users to print the content of the current webpage. Here's an explanation:
The print() method is used to initiate the printing of the current webpage, including text, images, visuals, and other content. It is typically triggered by a user action, such as clicking a print button.
The printing operation occurs when the browser finishes rendering the page. If the print-related dialog doesn't open during page loading, it may not open afterward. It's crucial to invoke the print method when the page is fully loaded to ensure a smooth printing experience.
The print()method is the part of window object, and it can be used to print content of a window. The information from the active window is printed using the print function. The print window appears, allowing the user to choose the necessary printing options.. This approach becomes blocked if the print method is invoked when the file is still not fully loaded.
Syntax:
window.print();
Parameter: There are no parameters required in this method.
The value returned: Nothing is returned.
Implementation Example
Create a button with an onClick event tied to the printPage() function to print a page. This button should activate when the page is printed. The printpage() function (in the script tag) will be invoked whenever the user hits the button. This method may contain some code that aids in printing the page. A dialogue window with the ability to save the file will show up.
Example: This instance shows how to output the present-day webpage in Javascript using the print() method.
Code:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript print() Method</title>
</head>
<body>
<h2>Tutorials and examples</h2>
<h5>Javascript print() Method</h5>
<p>
An instructional portal for technical subjects
There are effective, thoughtful, and clearly described technological and computer articles.
</p>
<button onclick="printPage()" style="color:white;
background-color: red;
font-weight: bold;">Click
</button>
<script>
function printPage() {
window.print();
}
</script>
</body>
</html>
Output:
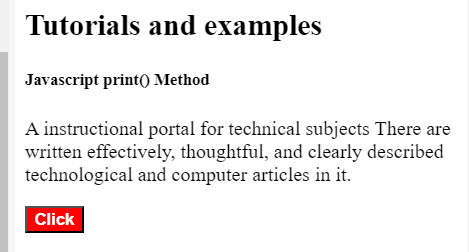
Making use of a print library
Here, we add the print JavaScript library file to our HTML. For printing the file, this file's custom method is called. Users can access to the language listed below to utilise this library's features.
Syntax:
<script rel = "stylesheet" href="CSS source url"/>
<script src = "javascript source url"> </script>
printJS('html','id');
Here, the individual print method is invoked in the subsequent syntax, and the necessary CSS and JavaScript files are supplied using the initial two structures.
Parameters:
- id: The attribute id is used to describe value of the HTML material's property to print.
- HTML - The kind of print material, Files or photos may also be included.
Example
We have loaded the necessary modules here. The print-only content ID is the first argument passed to the customized function in a library when the user hits the button. The user is presented with the printing box.
Code:
<html>
<head>
<script src="https://printjs-4de6.kxcdn.com/print.min.js"></script>
<link rel="stylesheet" href="https://printjs4de6.kxcdn.com/print.min.css" />
</head>
<body>
<h2>printing a page using JSP</h2>
<div id="libInp">
<p>Tutorials</p>
<p>and</p>
<p>examples</p>
</div>
<div id="libBtnWrap">
<h4>Click on the botton</h4>
<button onclick="libPrint()">Print</button>
</div>
<p id="libOp"></p>
<script>
var libInpEl = document.getElementById("libInp");
var libOutEl = document.getElementById("libOp");
var libBtnWrapEl = document.getElementById("libBtnWrap");
function libPrint() {
libOutEl.innerHTML = "Printing the document...";
libBtnWrapEl.style.display = "none";
printJS('libInp', 'html');
}
</script>
</body>
</html>
Output:
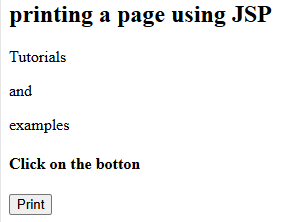