Component Design in Software Engineering
Component design is a cornerstone concept in software engineering, shaping how software systems are conceived, built, and maintained. In today's dynamic and ever-evolving digital landscape, where software plays a pivotal role across industries, understanding the significance of component design is paramount. This article delves into component design, elucidating its vital role in software development. We will explore the fundamental principles underpinning component design, its far-reaching benefits, developers' challenges, essential best practices, and emerging trends in this critical domain of software engineering. Welcome to a comprehensive journey into the heart of efficient and effective software design.
Understanding Component Design
We encounter a fascinating concept in software engineering called "software components." These components are akin to the building blocks of software, each one encapsulating a specific piece of functionality, data, or service. Think of them as the building materials that software developers use to construct complex applications.
The significance of component-based design cannot be overstated. It is the cornerstone upon which scalable, maintainable, and efficient software systems are crafted. When we delve into the realm of component design, we embark on a journey that offers a multitude of advantages to the world of software development.
First and foremost, component-based design promotes the invaluable attribute of reusability. Well-structured components are like treasures that can be utilized across various projects, sparing developers the redundancy of reinventing the wheel and saving precious time and effort. This reusability saves resources and ensures consistency, as a proven component can be relied upon to perform its designated function consistently.
Moreover, components play an essential role in the grand scheme of modular software architecture. They empower the creation of systems composed of distinct, interchangeable parts. This modularity unleashes the power of flexibility, making it a breeze to adapt and extend the software as needs evolve or novel features are introduced. It also simplifies the often intricate tasks of debugging and maintenance by isolating issues within individual components.
In essence, component-based design is the bedrock of robust, adaptable, and scalable software systems, making it an indispensable practice in the toolkit of modern software engineering.
Key Principles of Component Design
Effective component design in software engineering is guided by fundamental principles that serve as the building blocks for creating robust and maintainable software systems. These principles, namely encapsulation, reusability, abstraction, and modularity, are essential in shaping the structure and functionality of software components.
1. Encapsulation
Encapsulation is akin to the concept of a "black box." It refers to bundling data (attributes) and the methods (functions) that operate on that data into a single unit—a component. By doing so, a component's internal details and complexities are hidden from the outside world. This provides several benefits:
- Information Hiding: Encapsulation allows developers to protect the integrity of a component's internal state. The internal workings of a component are hidden, preventing unintended interference from external code.
- Ease of Maintenance: Changes to the internal implementation of a component can be made without affecting other parts of the system that rely on it. This promotes ease of maintenance and code stability.
2. Reusability
Reusability is a cornerstone of component design. It emphasizes the creation of components that can be reused across different parts of a software system or even in entirely different projects. When components are designed with reusability in mind, it brings several advantages:
- Efficiency: Reusable components save development time and effort by eliminating the need to recreate similar functionalities from scratch.
- Consistency: Reusing well-tested and proven components ensures consistency in behavior and reduces the likelihood of errors or bugs.
3. Abstraction
Abstraction simplifies complex systems by focusing on essential properties and ignoring non-essential details. In component design, abstraction involves defining a component's interface without exposing its internal workings. This allows developers to work with higher-level concepts, facilitating easier understanding and maintenance of the software.
4. Modularity
Modularity is the division of a software system into smaller, self-contained units or modules components in this context. Each module has a specific responsibility and interacts with others through well-defined interfaces. Modularity promotes:
- Scalability: Adding new features or functionalities becomes more manageable as new modules can be integrated without affecting existing ones.
- Concurrent Development: Different teams or developers can work on separate modules simultaneously, fostering parallel development and collaboration.
Benefits of Component Design
Component-based design offers many advantages in software engineering, making it a preferred approach for building complex software systems. Here are four key benefits, along with real-world examples that highlight their significance:
1. Maintainability
Maintaining software can be daunting, but component-based design eases this burden. Updates or fixes can be localized without impacting the entire system when each component is self-contained and adheres to specific rules. For instance, plugins act as components in the widely-used content management system (CMS) WordPress. Developers can update a plugin to enhance security or add new features without disrupting the core CMS functionality.
2. Scalability
As software requirements grow, scalability becomes crucial. Component-based design allows for easy scaling by adding or replacing components. In cloud computing, microservices exemplify this benefit. Each microservice handles a specific task, such as user authentication or payment processing. When demand increases, you can scale individual microservices independently to handle more requests without overhauling the system.
3. Reusability
Components designed for reuse save time and effort. Take the example of the React JavaScript library. React components encapsulate UI elements and logic. Developers can create and use a button component across various application parts, ensuring consistent styling and behavior.
4. Collaboration
In collaborative software development, component-based design fosters efficient teamwork. Multiple teams or developers can work on separate components concurrently. A real-world example is the Linux operating system. Thousands of developers worldwide contribute to different parts of the kernel while ensuring that their changes do not disrupt other components.
Challenges in Component Design
While embracing component-based design yields numerous benefits, it is essential to acknowledge that it brings forth its hurdles, requiring software engineers to navigate them skilfully. In the following sections, we delve into three prevalent challenges: interoperability concerns, version control intricacies, and the complexities of testing, while also presenting effective strategies for tackling these obstacles.
1. Interoperability Issues
Interoperability challenges arise when different components must work together seamlessly, especially in complex systems with diverse technologies. To mitigate these challenges, adhere to well-defined interfaces and standards. Use technologies like API gateways to mediate between components with incompatible interfaces. Additionally, thorough documentation and communication among development teams can help identify and address interoperability issues early in development.
2. Version Control
Managing versions of individual components within a larger system can be complex. Implement versioning schemes for components, such as Semantic Versioning (SemVer), to overcome version control challenges. Employ version control systems like Git to track changes in component code and ensure backward compatibility whenever possible. Continuous integration and automated testing can help identify compatibility issues early.
3. Testing Complexities
Testing components in isolation might uncover only some potential issues when interacting within a larger system. To address testing complexities:
- Perform comprehensive integration testing.
- Create test harnesses to simulate real-world interactions between components.
- Implement automated testing to validate component behavior and use tools like mocking frameworks to simulate external dependencies.
Tools and Technologies
Many tools and technologies in component design empower software engineers to create efficient and maintainable software systems. Notable among these are:
1. Dependency Injection Frameworks
Dependency injection is a crucial component design concept. Frameworks like Spring (for Java) and Dagger (for Android) facilitate the implementation of this principle, allowing components to receive their dependencies rather than creating them internally. This promotes flexibility and testability.
2. Component-Based Frameworks
Frameworks such as Angular and React are exemplary models of component-based design. They provide a structured approach to building user interfaces by breaking them into reusable components, each encapsulating its logic and view.
3. Design Patterns
Design patterns like Singleton and Factory are pivotal in component design. Singleton ensures the existence of a single instance of a class, promoting resource efficiency. Factory simplifies object creation, enhancing the flexibility of component instantiation.
These tools and technologies serve as essential building blocks in the toolkit of software engineers, facilitating the creation of modular, maintainable, and scalable software systems through effective component design.
Best Practices
Adhering to best practices is paramount for achieving optimal results when engaging in component design. Here are key guidelines to consider:
1. Clear Naming Conventions
Employ descriptive and consistent naming conventions for components and their elements. Clarity in nomenclature aids in understanding and maintaining the codebase.
2. Documentation
Thoroughly document components, their interfaces, and usage. Well-structured documentation facilitates collaboration and ensures the longevity of the software.
3. Separation of Concerns
Maintain a clear separation of concerns within components. Each component should have a single, well-defined purpose, promoting modularity and ease of maintenance.
4. Regular Code Reviews
Foster a culture of regular code reviews within the development team. Reviews help identify issues, ensure adherence to best practices, and encourage knowledge sharing.
These best practices are a foundation for crafting efficient, maintainable, collaborative software components that stand the test of time.
Case Studies
Netflix
A global streaming giant, Netflix leverages a sophisticated component-based architecture for its user interface. The company's UI comprises reusable components that encapsulate functionalities like video playback, recommendation algorithms, and user profiles. This approach allows Netflix to roll out new features rapidly and maintain a consistent experience across various devices. Their component-based design has contributed to their global success and ability to seamlessly adapt to changing viewer preferences.
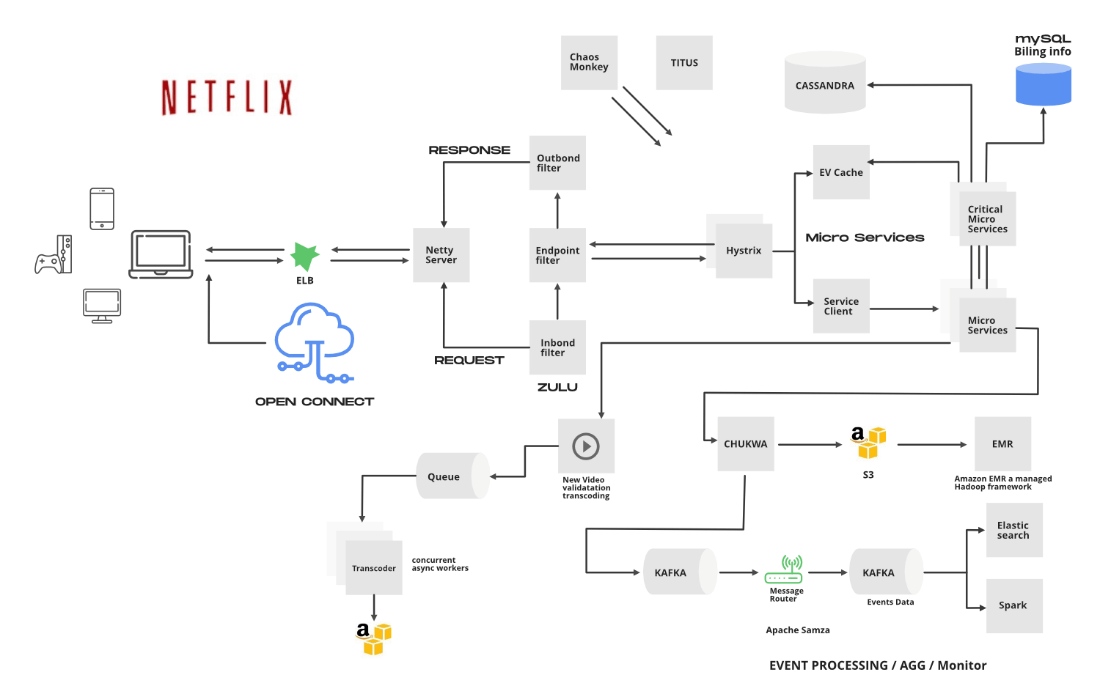
Fig: Component design for Netflix
Airbnb
Airbnb, a leader in the vacation rental industry, employs component-based design principles for its web and mobile applications. Their design system, known as "DLS" (Design Language System), consists of reusable components such as navigation bars, search filters, and booking modules. This approach streamlines development, ensures a consistent user experience, and enables Airbnb to innovate quickly. Component design has been pivotal in maintaining Airbnb's position as a market leader in the travel industry.
Conclusion
To sum it up, component design is the bedrock of contemporary software engineering. Embracing its guiding principles encapsulation, reusability, abstraction, and modularity - equips developers to construct scalable and maintainable software systems adaptable to the dynamic demands of today's technology-driven world.