Node.js util.formatWithOptions() Method
Node.js util.formatWithOptions() Method
Node.js is a versatile framework for building JavaScript server-side applications. Among its many utilities is the util module, which provides several helpful features. Among these is util.formatWithOptions(), a little-known but highly useful method. This method enhances string formatting capabilities by providing more control over parameters and output formats. This article will examine the util.formatWithOptions() method, along with its syntax, use, and practical applications. It will also provide instances to illustrate its benefits.
What is the Node.js util.formatWithOptions() function?
One function included in the Node.js util module is util.formatWithOptions() function. This function provides options and parameters so that developers may format strings more sophisticatedly. When information logging or debugging is involved, this method is very useful because total control over the output format is crucial.
Syntax of util.formatWithOptions():
It has the following syntax:
util.formatWithOptions(inspectOptions, format[, ...args])
Parameters:
An object that specifies parameters for the util.inspect method is called inspectOptions. These settings manage the formatting of objects and other complicated data types.
- Format: A string with one or more placeholder characters.
- ...args: Values to be entered into the format string's placeholders.
Understanding Placeholders
There may be placeholders in the format string; these are replaced with the values from...args. The placeholders resemble those found in the sprintf function in other languages and the printf function in C. Typical placeholders consist of:
- %s: String.
- %d: Number (integer or floating point).
- %i: Integer.
- %f: Floating point number.
- %j: JSON.
- %o: Object.
- %%: Literal % character.
Inspect Options
The format of objects and other complicated data types is set by the inspectOptions option. It is an object whose attributes may adjust to personalize the result. Among the essential characteristics are:
- ShowHidden: It indicates whether an object's hidden (non-enumerable) attributes are displayed.
- Depth: It indicates the level of recursion for nested items.
- Colors: In order to make the output easier to see at the terminal, if true, add ANSI color codes to it.
- CustomInspect: Custom inspect functions declared on objects are ignored if customInspect = false.
Use Case Examples
let's examine a few instances to get a sense of how util.formatWithOptions() actually functions.
Basic Example:
const util = require('util');
const optns = { colors: true };
const str = util.formatWithOptions(optns, 'Hello, %s! You have %d new messages.', 'Alice', 5);
console.log(str);
Output:

Explanation:
In this example, the format string contains two placeholders: %s for a string and %d for a number.
Using Inspect Options
const util = require('util');
const obj = { name: 'Alice', age: 30, hobbies: ['reading', 'biking'] };
const optns = { colors: true, depth: 2 };
const str = util.formatWithOptions(optns, 'User info: %o', obj);
console.log(str);
Output:
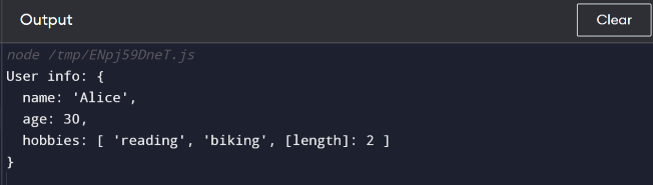
Explanation:
Here, the inspect options include colors and depth. The depth option controls how deeply nested objects are formatted. The output will be colorized, and the object structure will be shown up to two levels deep.
Handling Circular References
Circular references in objects can cause problems in formatting. The util.formatWithOptions() function handles these gracefully.
const util = require('util');
const obj = {};
obj.self = obj;
const options = { depth: null };
const str = util.formatWithOptions(options, 'Circular reference: %o', obj);
console.log(str);
Output:
In this case, the object obj references itself. The method will output a string that indicates the circular reference, avoiding an infinite loop or crash:

Practical Applications
The util.formatWithOptions() function can be particularly useful in several scenarios:
1. Enhanced Logging
When logging information in a Node.js application, it is often helpful to format the output for readability. By using inspectOptions, developers can include hidden properties, control the depth of object inspection, and add colors to the output.
const util = require('util');
const obj = {
user: 'Alice',
notifications: {
messages: 5,
alerts: 2
},
preferences: {
theme: 'dark',
layout: 'grid'
}
};
const optns = { colors: true, depth: 2 };
const msg = util.formatWithOptions(optns, 'Log entry: %O', obj);
console.log(msg);
Output:
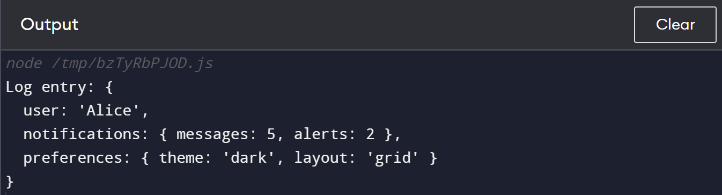
2. Debugging
Having detailed and formatted output of variables can be crucial during debugging. The util.formatWithOptions() function provides a way to inspect variables deeply, making it easier to understand their structure and content.
const util = require('util');
const obj = {
user: 'Alice',
notifications: {
messages: 5,
alerts: 2
},
preferences: {
theme: 'dark',
layout: 'grid'
},
hidden: Symbol('hiddenProperty')
};
const optns = { showHidden: true, depth: null, colors: true };
console.log(util.formatWithOptions(optns, 'Debug info: %O', obj));
Output:
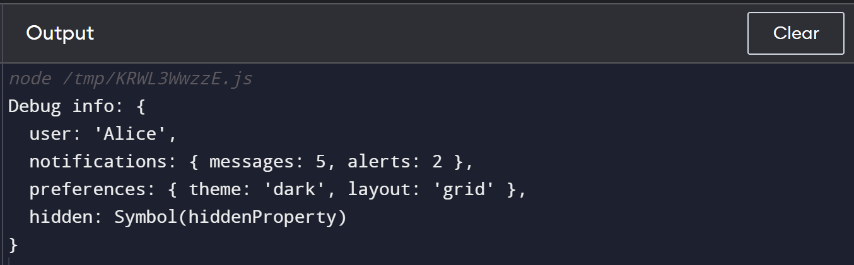
3. Custom Error Messages
When throwing errors, formatting the error message can help convey more context.
const util = require('util');
const inp = {
username: 'Alice',
age: 30,
preferences: {
theme: 'dark',
notifications: true
}
};
const options = { depth: 2 };
const msg = util.formatWithOptions(options, 'Invalid input: %O', inp);
console.error(msg);
Output:
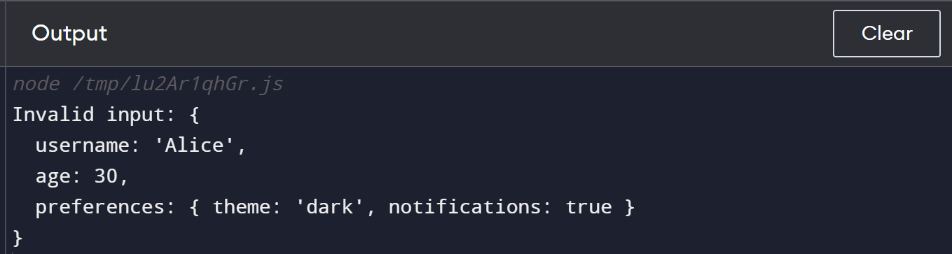
Explanation:
In this example, the error message includes a formatted representation of the invalid input, which can be very helpful for debugging.
Conclusion:
In conclusion, the util.formatWithOptions() method in Node.js is a powerful tool for formatting strings, especially when dealing with complex data structures. By leveraging inspectOptions, developers can gain fine-grained control over how objects and other types are displayed. This method proves invaluable in logging, debugging, and creating informative error messages. While it may not be as commonly used as other methods in Node.js, understanding and utilizing util.formatWithOptions() can significantly enhance the readability and maintainability of your code.