Top-down and Bottom-up Approach in Data Structure
Both top-down and bottom-up approaches to information processing and knowledge organization are employed in many domains, such as software, management and organization, and scientific and humanistic ideas (see systemic). In actuality, they might be viewed as ways of thinking, instructing, or leading.
A top-down technique involves disassembling a system in reverse engineering to obtain insight into its structural components. It is also referred to as stepwise design, stepwise refinement, and, in certain situations, as a synonym for decomposition. A top-down method creates a system overview that describes any first-level subsystems but does not go into depth. Then, until the specification as a whole has been simplified to base components, each subsystem is improved in even more depth, perhaps at several more subsystem levels.
Black boxes are frequently used to specify top-down models, which facilitate manipulation. However, black boxes might not provide enough detail to test the model realistically or might not be able to explain basic principles. A top-down method begins with the broad picture and works its way down to more manageable parts.
Piecing together systems to create more complex systems making the initial systems constituents of the emerging system is known as a bottom-up strategy. Bottom-up processing is a sort of information processing that builds perceptions by using input coming in from the outside world. According to cognitive psychology theory, information travels from the "bottom" (sensory input) into the eyes. The brain then processes it into a picture that can be evaluated and identified as a picture (output that is "created up" from the processor to final cognition).
A bottom-up method begins with a detailed specification of each of the system's component basic parts. After that, these components are connected to create bigger subsystems, which are connected one more time sometimes across many levels to build a whole top-level system. This tactic frequently has the appearance of a "seed" model, in which things start simple but gradually become more intricate and comprehensive. However, "organic strategies" could produce a jumble of components and subsystems that are designed separately and optimized locally rather than serving a universal goal.
Design and Development of Products
Designers and engineers use both top-down and bottom-up methods while creating new products. When pre-existing or off-the-shelf components are chosen and included in the product, the bottom-up methodology is applied. An illustration would be to choose a certain fastener, like a bolt, and then build the receiving elements such that the fastening fits correctly. A bespoke fastener would be made in a top-down manner so that it would fit correctly in the receiving pieces. To put things in perspective, a more top-down approach is used, and nearly everything is specially created for a product, like a spacesuit that has more stringent criteria (such as weight, shape, safety, and environment).
The creator of the Pascal programming language, Niklaus Wirth, is also credited with writing the seminal work Program Development by Incrementally Refinement. Top-down programming was different from what Niklaus Wirth espoused; as he went on to invent languages like Oberon and Modula, where one could specify a module before having knowledge about the complete program specification. Up until the late 1980s, software engineers preferred top-down methodologies. Object-oriented programming helped to illustrate that top-down and bottom-up development could both be used in tandem.
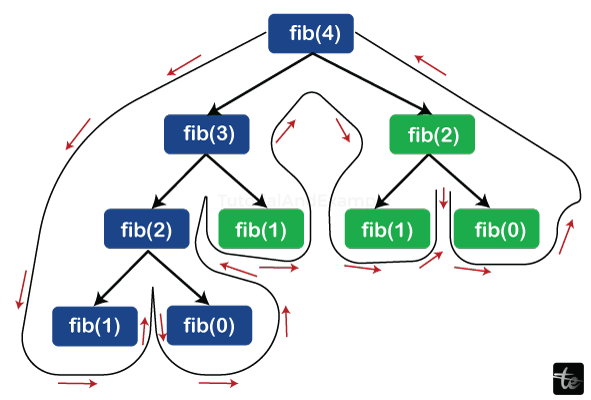
Contemporary software design methodologies frequently combine top-down and bottom-up techniques. While a top-down approach is theoretically possible only if one has a thorough grasp of the entire system, most software projects try to utilize some portion of the current code. Existing modules imbue designs with a bottom-up approach.
How to Program?
The foundation of classic procedural languages is the top-down programming method, in which big elements are first specified and then broken down into progressively smaller bits. Using top-down programming techniques, a program is written by naming all of the key functions it will require in a main process. The procedure is then repeated once the programming team has examined the specifications for each of those functions.
Eventually, these divided subroutines will carry out tasks that are straightforward enough to be programmed succinctly and easily. Once each of the several subroutines has been written, the program is prepared for testing. Lower-level tasks can be self-contained if the application's high-level architecture is defined.
A bottom-up method begins with a detailed specification of each of the system's basic parts. These components are then connected to create bigger subsystems, which are connected to one another sometimes at many levels to build a top-level system in its entirety. This tactic frequently resembles the "seed" approach, in which things start off tiny and gradually become more intricate and comprehensive. Computer programs and applications are designed using "objects" according to the object-oriented programming (OOP) paradigm.
Software Development in Computer Science
Both top-down and bottom-up techniques are important in the software process of development.
Top-down strategies place a strong emphasis on preparation and thorough system comprehension. It is a built-in fact that coding can start once the blueprint of at least a portion of the system has been completed to a suitable degree of detail. When using top-down methods, the module is replaced with the stubs. However, these wait to test a system's final functioning components until the major design is finished.
The bottom-up approach places a strong emphasis on early testing and coding, which may start as soon as the first module is described. However, this method has the danger that modules might be programmed without a clear understanding of how they connect to other system components and that connecting them might be more difficult than initially believed. The ability to reuse material is one of the key advantages of a bottom-up strategy.
In the 1970s, IBM engineers Harlan Mills as well as Niklaus Wirth supported top-down design. In order to verify his practical, structured programming notions, Mills worked on an automated New York Times cemetery index project in 1969.
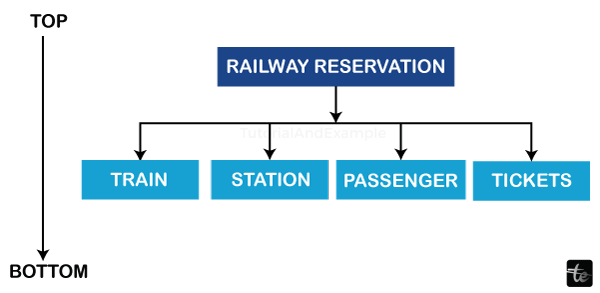
In mechanical engineering, users may design things as separate parts that are then assembled to make assemblies, much like Lego construction, using software applications like Pro/ENGINEER, Solidworks, and Autodesk Inventor. This is known as "piece-part design" in engineering.
The Use of Nanotechnology
There exist two methodologies for product manufacturing: top-down and bottom-up. The Foresight Institute coined these phrases in 1989 to distinguish either molecular manufacturing, which produces big, atomically accurate products in mass quantities, or traditional manufacturing, which may manufacture massive, non-atomically precise objects in mass quantities. While top-down methods aim to produce nanoscale devices by employing bigger, externally directed ones to drive their assembly, bottom-up approaches aim to have smaller (typically molecular) constituents built upward into more sophisticated assemblies.
Either strategy may be used to create some useful nanostructures, including silicon nanowires, with the chosen processing techniques determined by the intended uses.
In a top-down technique, materials are cut, milled, and shaped into the required shape and order using externally controlled tools, much like in a typical workshop or microfabrication process. This group includes micropatterning methods including photolithography and inkjet printing. One innovative top-down secondary method for engineering nanostructures is vapour treatment.
On the other hand, bottom-up techniques rely on positional assembly or (a) self-organization or self-assembly of single-molecule components into a usable conformation using the chemical characteristics of individual molecules. These methods make use of the ideas of molecular recognition and molecular self-assembly.
Also see Chemistry of the supramolecular. In general, bottom-up systems can build devices more quickly and affordably than top-down methods by producing them in parallel. However, their effectiveness may diminish as the number of components and size of the intended assembly increase.
Psychology and Neuroscience
These phrases are also used to explain the flow through which data is processed in the fields of neuroscience, cognitive neuroscience, and cognitive psychology. In general, higher cognitive processes—which have access to more information from outside sources are thought of as top-down, whereas sensory input is thought of as bottom-up. A top-down process can be defined by a high degree of direction to sensory processing by greater cognition, such as objectives or aims. In contrast, a bottom-up process lacks higher-level directing in sensory processing (Biederman, 19).
As per Charles Ramskov's teaching notes for college, the top-down method entails a constructive and active process of perception, according to Rock, Neiser, and Gregory. Also, it is a method that results from the combination of expectations, internal assumptions, and stimulus rather than being delivered directly by stimulus input. "Perception becomes a top-down strategy when a stimulus is made short, and sharpness is uncertain, thus gives a vague stimulus," states theoretical synthesis.
On the other hand, psychology describes bottom-up processing as a method where the individual components develop toward the total. Gibson, a supporter of the bottom-up method, asserts that visual perception is a process that requires information from the proximal stimulus generated by the distal stimuli, as per Ramskov. Bottom-up processing, according to theoretical synthesis, happens "when an event is made available long and precisely enough."
Public Well-being
In public health, both top-down and bottom-up methods are employed. Numerous top-down initiatives exist, frequently overseen by major IGOs or governments. Many of these focus on eradicating a particular illness or condition, like smallpox or HIV. Numerous little NGOs founded to provide local people with healthcare are examples of bottom-up initiatives. However, many initiatives aim to combine the two strategies. For example, the Carter Center's international program for the eradication of guinea worms, which is a single disease, has trained numerous local volunteers, increasing bottom-up capacity. Similarly, international initiatives for primary healthcare, sanitation, and hygiene have also involved training local volunteers.
Because they largely rely on sensory input, some cognitive functions, like quick visual recognition or fast reflexes, are regarded as bottom-up processes; in contrast, top-down processes, including motor control as well as focused attention, are goal-directed. In terms of neurology, area V1 and other brain regions mostly have bottom up connections. Some regions, like the fusiform gyrus, are thought to have a top-down effect because they receive information from higher brain regions.
One example is the study of attention to visuals. A brightly coloured plant in a field may catch your eye if its colour or form makes it stand out from the background. You did not need to know anything about the flower in order for you to pay attention to it; the external stimulation was adequate in and of itself. This is known as a bottom-up approach. Compare this to a scenario when you are trying to find a flower. What you are searching for is represented by what you have. The item you are seeking is conspicuous when you see it. An illustration of the application of top-down information is this.
Two distinct ways of thinking about cognition are identified. "Top–down" (also known as "big chunk") refers to the inspirational or the individual who has an overall and bigger-picture view. These individuals concentrate on the larger picture and infer the supporting elements from it. "Bottom–up" (also known as "small chunk") perception is similar to concentrating more on the details than the overall scene. "Seeing the wood alongside the trees" is a phrase that alludes to these two ways of thinking.
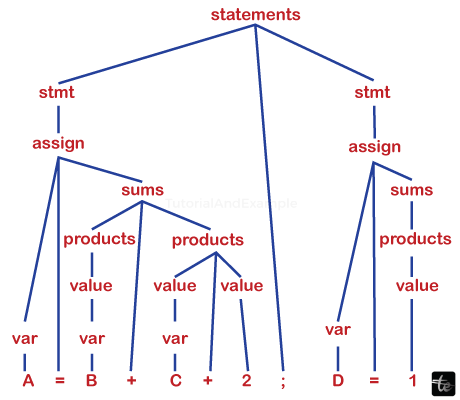
Research on response selection and task switching demonstrates the distinctions between the two processing modalities. The attention side of things, such as task repetition, is the main emphasis of top-down processing (Schneider, 2015). Finding the same thing again is an example of item-based learning that is the focus of bottom-up processing (Schneider, 2015). Schneider (2015) discusses the implications for comprehending attentional regulation of choice of reaction in conflict contexts.
Control and Structure
A "top–down" strategy is one in which an administrative choice maker or another high-ranking individual makes choices on how to proceed. Lower echelons of the hierarchy get this strategy through their authority and are, in some way, constrained by them. For instance, a hospital administrator seeking to enhance the facility could determine that a significant adjustment (such as introducing a new program) is required and then employ a deliberate strategy to communicate the changes to the front-line workers.
Changes that are implemented in a bottom-up manner start at the local level, where individuals collaborate in a flat hierarchy to conclude their combined efforts. Action taken by a group of activists, kids, or victims of an incident is referred to as a "bottom–up" choice. "An evolutionary change methodology that represents a natural phenomenon created and upheld predominantly by frontline workers" is one way to conceptualize a bottom-up strategy.
Top-down methods include advantages such as excellent overviews of higher levels and efficiency, as well as the ability to absorb external influences. Conversely, lower levels may find it challenging to embrace reforms if they are thought to be imposed "from above" (Bresser-Pereira, Maravall, and The Polish Politician 1993). Research indicates that this is accurate independent of the changes made (e.g., Dubois 2002). More innovation and a deeper understanding of what is required at the bottom are made possible by a bottom-up strategy. There may be a third combined strategy for transformation, according to additional findings.