Python List Files in a Directory Recursively
In Python, obtaining a complete list of files and subdirectories within a given directory and all subdirectories, irrespective of their level of nesting, is referred to as listing files in a directory recursively. Recursive listing is mainly used to access and handle files in several directory layers
effectively.
We can use various techniques to list files in a directory recursively. You can select the best strategy according to your requirements and preferences.
- You may communicate with the operating system using a number of the functions provided by the Python os module. One of these methods returns a list of the files and folders in a specified path, os.listdir().
- You need to develop a unique recursive method that iterates over each directory and uses os.listdir() on its contents to accomplish recursive listing. This strategy calls for more physical labor but may work for straightforward scenarios.
- Another method to list files in Python is the pathlib. The pathlib module is a more recent (added in Python 3.4) addition to Python, offering a more practical and object-oriented approach to interact with file paths. The Path class, which represents file and directory paths, is one of its offerings. Methods like rglob(), which lets you list files recursively from a given directory and its subdirectories, are available in the Path class. This strategy is typically seen as being more elegant and readable.
- Python has the required tools to recursively list files in a directory, whether you select the classic os module or the more contemporary pathlib module. Generally speaking, people prefer the pathlib technique because it is simpler and has a cleaner syntax, although both may do the same task successfully.
Using Python OS Module
The Python OS module offers a platform-independent means of interacting with the operating system, and it may be used to recursively list files in a directory. To specifically go all over the directory and its subdirectories, apply the os.walk() method.
How to Import the os module: To use its functionalities, you must first import it.
- Utilise os.walk(): The os.walk() method creates the file names in a directory tree rooted in a given directory. To access each directory, it provides a generator that produces a tuple containing three values. The directory's path, a list of the directory subdirectories, and a list of its present files are all included in the tuple.
- By os.walk(), the generator will iterate using this method. You'll get the current directory path, a list of subdirectories in the directory, and a list of files for each iteration.
- After that, you can do such according to your needs. You could print their names or work on them in some other way.
Example Code Using the OS Module
import os
#import the os module to use it
def list_files_recursive(directory):
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
full_path= os.path.join( dirpath, filename)
print(full_path)
# This is just the sample code you need to replace the directory path with the actual path
yourdirectorypath = '/path/to/required/directory'
list_files_recursive(yourdirectorypath)
Output:
Let the directory look like
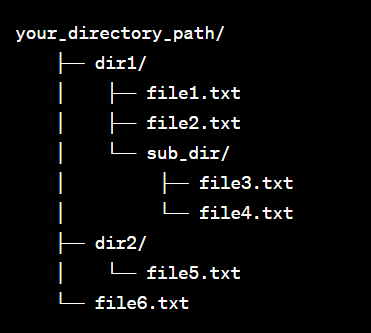
Then the output will be
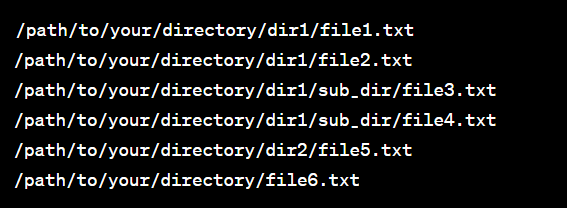
Explanation:
List_files_recursive (directory) is a function defined in the Python code to display a list of all files in a directory and all of its subdirectories. The method travels across the directory tree based on the provided directory using the os.walk() function using the os package. The method collects a tuple with the present directory's path (dirpath) and a list of its subdirectories (dirnames), and a list of its files (filenames) for each directory that was visited during the traverse.
The code then loops over each directory's list of filenames, creating each file's path by merging the dirpath and corresponding filename using os.path.join(). It displays each file's complete path in the directory tree. The actual path to the directory you wish to list must be replaced with the your_directory_path variable before using the function. When the code is run, all files existing in the specified directory and its subdirectories will have their complete paths printed out.
Using Python Pathlib Module
Pathlib is a Python module that was first made available in Python version 3.4 and offers a more user-friendly and object-oriented approach to interact with file paths and conduct filesystem operations. Since it is a standard library component, It does not require any installation, but you need to upgrade the existing Python to the 3.4 or above version.
For managing filesystem paths and operations before the introduction of pathlib, Python programmers mostly used the os and os.path modules. These modules still operate but may produce less understandable code since they manipulate paths via strings.
Path objects that stand for filesystem paths may be created with pathlib. Working with files and directories is made simpler by the many techniques and characteristics that these objects contain.
Example:
from pathlib import Path
def find_python_files_recursive(directory_path):
# Create a Path object representing the specified directory
directory = Path(directory_path)
# Initialize an empty list to store the found Python files
python_files = []
# Use the rglob method to search for files with the .py extension recursively
for file_path in directory.rglob('*.py'):
# Add the file path to the python_files list
python_files.append(file_path)
# Return the list of Python files found in the directory and its subdirectories
return python_files
# Call the function with the current directory (change '.' to any other directory path if needed)
python_files_list = find_python_files_recursive('.')
print("List of Python files found:")
for file_path in python_files_list:
print(file_path)
Output:

Explanation:
We start by importing the Path class from the pathlib library. We may interact with filesystem paths in a more object-oriented way because of this class.
- Next, we define the directory_path-inputted find_python_files_recursive function. This method will search the supplied directory and subdirectories recursively for Python files.
- We build a Path object named directory by supplying the directory_path to the Path constructor within the method. This makes it easier for us to deal with the designated directory.
- The file paths of all the Python files found in the directory and its subdirectories are initialized in an empty list named python_files.
- We employ the directory object's rglob function. The glob technique, used for pattern matching in directories, is available in a recursive form called rglob. To match all files with the.py extension (i.e., Python files), we provide the pattern '*.py'.
- Each file path the rglob function gives is iterated through in the for loop. It adds the file_path to the list of Python files for each one it discovers.
- The method returns the python_files list after the loop, containing the file paths to every Python file identified in the directory and its subdirectories.
- Then, using the current directory ('.'), we execute the find_python_files_recursive function and save the output in the variable python_files_list. The path to every Python file is printed when we display a message and cycle over the list.
Advantages of Using Pathlib for Recursive File Listing
- Simplicity and Readability: Pathlib offers a more natural and object-oriented method of working with filesystem paths than the typical string-based manipulation using the os module. The code gets more understandable, which facilitates understanding and maintenance.
- Simple Syntax: With the help of pathlib, file operations may be carried out with less code. Without the need for numerous function calls, handling file paths and carrying out different actions is simple because of the methods and properties of the Path class.
- Object-Oriented Interface: Pathlib allows you to communicate with filesystem paths as objects (instances of the Path class). This method supports a more object-oriented approach to programming, which makes the code simpler to understand and manage.
- Path manipulation: The Path class offers several ways to handle paths, such as connecting paths, addressing symlinks, obtaining the parent directory, and more. These techniques simplify typical path-related tasks.
- Exception Handling: Pathlib generates exceptions with more informative error messages, simplifying debugging problems with file paths and operations.
- No need for os.path methods: Because pathlib provides these features directly, there is no longer a need to employ os.path module methods like os.path.join, os.path.exists, and os.path.abspath.
- Recursive File Operations: The rglob function in pathlib allows recursive file operations, as seen in the code samples. This feature makes it simple to search for files in a directory and its subdirectories with only one method call.
- Seamless Conversion: Since Path objects can be conveniently transformed to and from strings, converting existing code that utilizes os.path to pathlib is relatively easy.